The largest element of the binary search tree must be in the right subtree of the root.
时间: 2024-06-04 15:07:10 浏览: 154
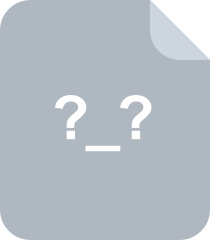
Binarysearchtree
That is correct. In a binary search tree, all elements in the left subtree of a node are less than the node itself, and all elements in the right subtree are greater than the node itself. Therefore, the largest element must be in the right subtree of the root, as there cannot be any elements greater than the root in the left subtree.
阅读全文
相关推荐
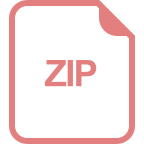

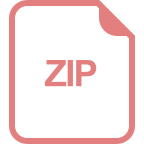
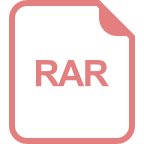
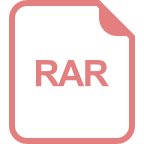
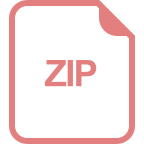
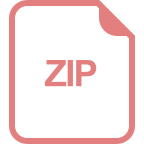
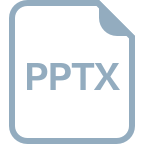
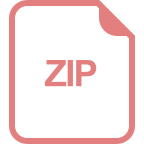
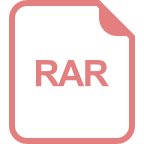
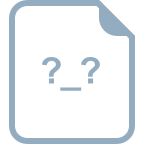


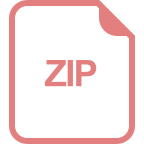