解释一下这段代码:public class HongBaoService extends AccessibilityService { public static HongBaoService mService; mService = this;}
时间: 2023-06-13 10:02:10 浏览: 52
这段代码定义了一个名为`HongBaoService`的类,并继承了`AccessibilityService`类。其中,`AccessibilityService`是一个Android系统提供的服务,可以帮助开发人员创建无障碍服务,使得用户可以更方便地使用应用程序。
在`HongBaoService`类中,定义了一个静态变量`mService`,并将当前对象`this`赋值给它。这样做的目的可能是为了在其他地方方便地访问`HongBaoService`实例对象。
需要注意的是,这段代码只是类的定义部分,没有在任何方法中被调用,因此可能存在语法错误或者逻辑错误。
相关问题
解释一下这段代码中的this是什么意思:public class HongBaoService extends AccessibilityService { public static HongBaoService mService; mService = this;}
在这段代码中,this是指当前类的实例对象。具体来说,在这个类中定义了一个静态变量mService,它的类型是HongBaoService,也就是当前类的类型。当创建一个HongBaoService类的实例对象时,通过this关键字获取到这个实例对象,并将其赋值给静态变量mService,使得其他地方可以通过mService来访问这个实例对象的属性和方法。
安卓service的多种用法 详细代码
1. 启动Service
启动Service是最常见的一种方式,通过Context的startService()方法启动Service,可以在后台执行一些耗时的操作,不会阻塞UI线程。例如:
创建一个MyService类,继承自Service:
```
public class MyService extends Service {
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
// 在这里执行耗时操作
return super.onStartCommand(intent, flags, startId);
}
@Nullable
@Override
public IBinder onBind(Intent intent) {
return null;
}
}
```
在Activity中启动Service:
```
Intent intent = new Intent(this, MyService.class);
startService(intent);
```
2. 绑定Service
绑定Service可以让Activity和Service之间建立联系,可以通过ServiceConnection获取Service实例,从而调用Service中的方法。例如:
创建一个MyService类,实现一个计算器功能:
```
public class MyService extends Service {
private final IBinder mBinder = new MyBinder();
public class MyBinder extends Binder {
MyService getService() {
return MyService.this;
}
}
@Override
public IBinder onBind(Intent intent) {
return mBinder;
}
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
}
```
在Activity中绑定Service:
```
private MyService mService;
private boolean mBound = false;
private ServiceConnection mConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName componentName, IBinder iBinder) {
MyService.MyBinder binder = (MyService.MyBinder) iBinder;
mService = binder.getService();
mBound = true;
}
@Override
public void onServiceDisconnected(ComponentName componentName) {
mBound = false;
}
};
@Override
protected void onStart() {
super.onStart();
Intent intent = new Intent(this, MyService.class);
bindService(intent, mConnection, Context.BIND_AUTO_CREATE);
}
@Override
protected void onStop() {
super.onStop();
if (mBound) {
unbindService(mConnection);
mBound = false;
}
}
// 调用Service中的方法
int result = mService.add(1, 2);
```
3. 前台Service
前台Service是一种可以让Service在通知栏显示的方式,可以让用户知道Service正在运行,避免被系统杀死。例如:
创建一个MyService类,启动前台Service:
```
public class MyService extends Service {
private static final int NOTIFICATION_ID = 1;
@Nullable
@Override
public IBinder onBind(Intent intent) {
return null;
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Notification notification = new NotificationCompat.Builder(this, "channel_id")
.setContentTitle("Service is running")
.setContentText("Service is running in foreground")
.setSmallIcon(R.drawable.ic_launcher_foreground)
.build();
startForeground(NOTIFICATION_ID, notification);
return START_STICKY;
}
}
```
在Activity中启动前台Service:
```
Intent intent = new Intent(this, MyService.class);
startService(intent);
```
4. IntentService
IntentService是一种特殊的Service,可以在后台执行一些耗时操作,不会阻塞UI线程。IntentService会自动停止,当所有的请求都处理完成后。例如:
创建一个MyIntentService类,继承自IntentService:
```
public class MyIntentService extends IntentService {
public MyIntentService() {
super("MyIntentService");
}
@Override
protected void onHandleIntent(Intent intent) {
// 在这里执行耗时操作
}
}
```
在Activity中启动IntentService:
```
Intent intent = new Intent(this, MyIntentService.class);
startService(intent);
```
5. JobIntentService
JobIntentService是一种特殊的Service,可以在后台执行一些耗时操作,不会阻塞UI线程。JobIntentService会自动停止,当所有的请求都处理完成后。与IntentService不同的是,JobIntentService会优先使用JobScheduler调度器,从而能够保证任务的执行顺序和优先级。例如:
创建一个MyJobIntentService类,继承自JobIntentService:
```
public class MyJobIntentService extends JobIntentService {
public static final int JOB_ID = 1;
@Override
protected void onHandleWork(@NonNull Intent intent) {
// 在这里执行耗时操作
}
public static void enqueueWork(Context context, Intent work) {
enqueueWork(context, MyJobIntentService.class, JOB_ID, work);
}
}
```
在Activity中启动JobIntentService:
```
Intent intent = new Intent(this, MyJobIntentService.class);
MyJobIntentService.enqueueWork(this, intent);
```
相关推荐
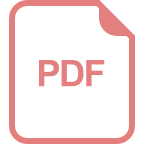
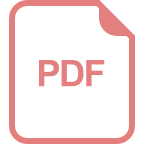
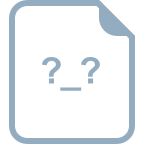







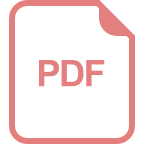
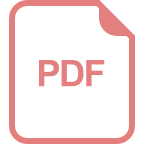
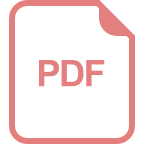
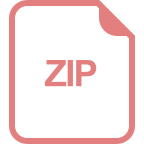
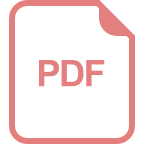
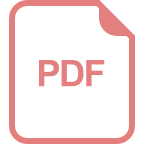
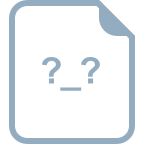