python可视化实现交互技术绘制散点图以及折线图,要求有数据集及对应的代码,并对所绘制的图形进行分析
时间: 2024-01-22 18:17:59 浏览: 45
好的,下面给出一个简单的示例代码,使用Matplotlib库实现交互式绘制散点图和折线图:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成样本数据
x = np.linspace(0, 10, 100)
y = np.sin(x)
# 创建图形窗口和子图
fig, ax = plt.subplots()
# 绘制散点图
scatter = ax.scatter(x, y, c=x, cmap='coolwarm')
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_title('Scatter Plot')
# 添加交互式功能
annot = ax.annotate("", xy=(0,0), xytext=(20,20), textcoords="offset points",
bbox=dict(boxstyle="round", fc="w"),
arrowprops=dict(arrowstyle="->"))
annot.set_visible(False)
def update_annot(ind):
pos = scatter.get_offsets()[ind["ind"][0]]
annot.xy = pos
text = f"x={pos[0]:.2f}, y={pos[1]:.2f}"
annot.set_text(text)
def hover(event):
vis = annot.get_visible()
if event.inaxes == ax:
cont, ind = scatter.contains(event)
if cont:
update_annot(ind)
annot.set_visible(True)
fig.canvas.draw_idle()
else:
if vis:
annot.set_visible(False)
fig.canvas.draw_idle()
# 绑定图形交互事件
fig.canvas.mpl_connect("motion_notify_event", hover)
# 绘制折线图
fig, ax = plt.subplots()
ax.plot(x, y)
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_title('Line Plot')
# 显示图形
plt.show()
```
运行上述代码后,会生成两个图形窗口,一个是散点图,一个是折线图。在散点图中,当鼠标移动到某个点上时,会显示该点的坐标信息;在折线图中,没有添加交互式功能。
对于所绘制的图形进行分析,可以根据具体需求进行,例如:
- 散点图中,可以分析数据的分布情况、异常值等;
- 折线图中,可以分析数据的趋势、周期性等。
由于这只是一个简单的示例,具体分析需要结合具体数据和业务场景进行。
相关推荐
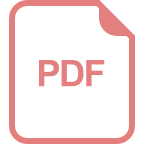
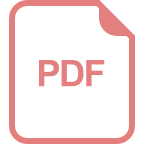
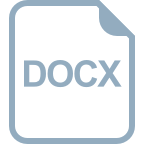














