51 模拟IIC
时间: 2023-09-28 16:11:58 浏览: 45
51 单片机可以通过软件实现 IIC 总线的模拟,以下是一个简单的示例代码:
```c
#include <reg51.h>
#define SCL P2_0
#define SDA P2_1
void delay_us(unsigned int us)
{
while(us--)
;
}
void iic_start(void)
{
SDA = 1;
delay_us(2);
SCL = 1;
delay_us(2);
SDA = 0;
delay_us(2);
SCL = 0;
delay_us(2);
}
void iic_stop(void)
{
SDA = 0;
delay_us(2);
SCL = 1;
delay_us(2);
SDA = 1;
delay_us(2);
}
unsigned char iic_read_byte(unsigned char ack)
{
unsigned char i, dat = 0;
SDA = 1;
for(i=0; i<8; i++)
{
dat <<= 1;
SCL = 1;
delay_us(2);
dat |= SDA;
SCL = 0;
delay_us(2);
}
SDA = ack;
SCL = 1;
delay_us(2);
SCL = 0;
delay_us(2);
return dat;
}
void iic_write_byte(unsigned char dat)
{
unsigned char i;
for(i=0; i<8; i++)
{
SDA = dat & 0x80;
dat <<= 1;
SCL = 1;
delay_us(2);
SCL = 0;
delay_us(2);
}
SDA = 1;
SCL = 1;
delay_us(2);
SCL = 0;
delay_us(2);
}
void iic_init(void)
{
SDA = 1;
SCL = 1;
delay_us(2);
}
int main()
{
unsigned char addr = 0x3C;
unsigned char data[2] = {0x00, 0x01};
iic_init();
iic_start();
iic_write_byte(addr << 1);
iic_write_byte(data[0]);
iic_write_byte(data[1]);
iic_stop();
return 0;
}
```
以上代码实现了向 IIC 地址为 0x3C 的设备写入数据 0x01,可以根据需要修改相应的地址和数据。需要注意的是,由于 51 单片机的处理速度有限,模拟 IIC 的时序可能不够准确,需要根据具体情况进行调整。
相关推荐
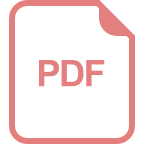














