<arrow/api.h>
时间: 2023-12-18 11:02:32 浏览: 130
这是 Apache Arrow 的头文件,Arrow 是一个跨语言的内存数据结构库,旨在提高大数据处理的效率和速度。该库支持多种编程语言,包括 C++, Java, Python 等。其中,<arrow/api.h> 是用于 C++ 的头文件,包含了 Arrow 的 C++ API。通过 Arrow,用户可以将数据在不同的系统之间高效地传输和共享。
相关问题
windowsApi
Windows API是Windows操作系统提供的一组核心API函数,可以用于开发Windows应用程序。这些API函数提供了许多功能,例如窗口管理、文件操作、网络通信等。使用Windows API可以让开发者更加灵活地控制Windows系统,实现更加复杂的功能。以下是一个使用Windows API创建一个简单窗口的示例:
```c
#include <windows.h>
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[] = TEXT("HelloWin");
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.style = CS_HREDRAW | CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH) GetStockObject(WHITE_BRUSH);
wndclass.lpszMenuName = NULL;
wndclass.lpszClassName = szAppName;
if (!RegisterClass(&wndclass))
{
MessageBox(NULL, TEXT("This program requires Windows NT!"),
szAppName, MB_ICONERROR);
return 0;
}
hwnd = CreateWindow(szAppName, // window class name
TEXT("The Hello Program"), // window caption
WS_OVERLAPPEDWINDOW, // window style
CW_USEDEFAULT, // initial x position
CW_USEDEFAULT, // initial y position
CW_USEDEFAULT, // initial x size
CW_USEDEFAULT, // initial y size
NULL, // parent window handle
NULL, // window menu handle
hInstance, // program instance handle
NULL); // creation parameters
ShowWindow(hwnd, iCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
HDC hdc;
PAINTSTRUCT ps;
RECT rect;
switch (message)
{
case WM_CREATE:
return 0;
case WM_PAINT:
hdc = BeginPaint(hwnd, &ps);
GetClientRect(hwnd, &rect);
DrawText(hdc, TEXT("Hello, Windows API!"), -1, &rect,
DT_SINGLELINE | DT_CENTER | DT_VCENTER);
EndPaint(hwnd, &ps);
return 0;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);
}
```
c++使用arrow库读取parquet文件
在C++中使用Arrow库来读取Parquet文件通常涉及以下几个步骤:
1. **安装依赖**:
首先,你需要在项目中添加Apache Arrow和相关的Parquet C++库作为依赖。这通常可以通过包管理器如`vcpkg`或直接从GitHub克隆仓库并手动构建。
2. **包括头文件**:
在源代码中,引入必要的头文件,比如`arrow/api.h`和`parquet/arrow/reader.h`。
```cpp
#include <arrow/api.h>
#include <parquet/arrow/reader.h>
```
3. **创建Schema**:
如果你知道数据的Schema(列名、类型等),可以使用Arrow Schema来描述它。如果没有,可以动态地解析Parquet元数据。
```cpp
arrow::schema schema = ...; // 从元数据获取或硬编码
```
4. **打开文件**:
使用`parquet::io::ReadableFile`打开Parquet文件,并创建一个`parquet::arrow::ColumnReader`实例。
```cpp
parquet::arrow::ColumnReader column_reader;
std::shared_ptr<parquet::io::ReadableFile> file = parquet::arrow::OpenInputFile("path_to_your_parquet_file", &column_reader);
```
5. **读取数据**:
使用`column_reader->ReadBatch()`方法逐批读取数据,直到文件结束。
```cpp
while (file->Tell() < file->Length()) {
std::unique_ptr<arrow::Array> batch = column_reader->ReadNext();
// 处理batch数据
}
```
6. **清理资源**:
当所有数据读取完成后,记得关闭文件和释放其他资源。
```cpp
file.reset();
```
阅读全文
相关推荐
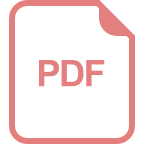
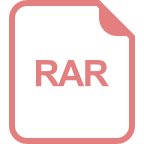
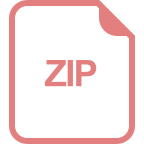
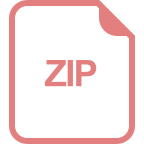
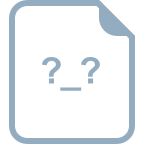
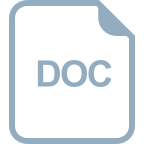
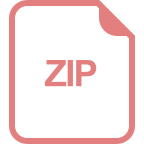
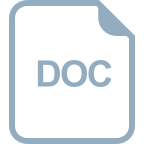
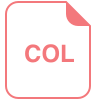
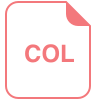
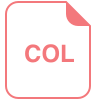
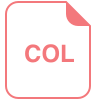
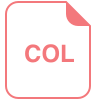
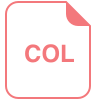
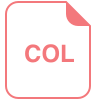
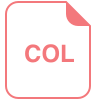
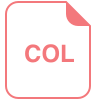