小车避障摄像头Python代码
时间: 2023-11-05 18:11:53 浏览: 118
以下是使用树莓派和OpenCV库实现小车避障的Python代码,其中摄像头连接到树莓派上,可以通过OpenCV库进行视频流的捕获和处理。
```python
import cv2
import numpy as np
import RPi.GPIO as GPIO
import time
# 声明GPIO口
GPIO.setmode(GPIO.BOARD)
GPIO.setwarnings(False)
Motor_A_EN = 11
Motor_A_Pin1 = 13
Motor_A_Pin2 = 15
Motor_B_EN = 12
Motor_B_Pin1 = 16
Motor_B_Pin2 = 18
# 设置GPIO口为输出
GPIO.setup(Motor_A_EN, GPIO.OUT)
GPIO.setup(Motor_A_Pin1, GPIO.OUT)
GPIO.setup(Motor_A_Pin2, GPIO.OUT)
GPIO.setup(Motor_B_EN, GPIO.OUT)
GPIO.setup(Motor_B_Pin1, GPIO.OUT)
GPIO.setup(Motor_B_Pin2, GPIO.OUT)
# 设置PWM频率
pwm_A_EN = GPIO.PWM(Motor_A_EN, 2000)
pwm_B_EN = GPIO.PWM(Motor_B_EN, 2000)
pwm_A_EN.start(0)
pwm_B_EN.start(0)
# 小车前进
def forward():
GPIO.output(Motor_A_Pin1, GPIO.HIGH)
GPIO.output(Motor_A_Pin2, GPIO.LOW)
GPIO.output(Motor_B_Pin1, GPIO.HIGH)
GPIO.output(Motor_B_Pin2, GPIO.LOW)
pwm_A_EN.ChangeDutyCycle(80)
pwm_B_EN.ChangeDutyCycle(80)
# 小车后退
def backward():
GPIO.output(Motor_A_Pin1, GPIO.LOW)
GPIO.output(Motor_A_Pin2, GPIO.HIGH)
GPIO.output(Motor_B_Pin1, GPIO.LOW)
GPIO.output(Motor_B_Pin2, GPIO.HIGH)
pwm_A_EN.ChangeDutyCycle(80)
pwm_B_EN.ChangeDutyCycle(80)
# 小车左转
def turn_left():
GPIO.output(Motor_A_Pin1, GPIO.HIGH)
GPIO.output(Motor_A_Pin2, GPIO.LOW)
GPIO.output(Motor_B_Pin1, GPIO.LOW)
GPIO.output(Motor_B_Pin2, GPIO.HIGH)
pwm_A_EN.ChangeDutyCycle(60)
pwm_B_EN.ChangeDutyCycle(60)
# 小车右转
def turn_right():
GPIO.output(Motor_A_Pin1, GPIO.LOW)
GPIO.output(Motor_A_Pin2, GPIO.HIGH)
GPIO.output(Motor_B_Pin1, GPIO.HIGH)
GPIO.output(Motor_B_Pin2, GPIO.LOW)
pwm_A_EN.ChangeDutyCycle(60)
pwm_B_EN.ChangeDutyCycle(60)
# 小车停止
def stop():
GPIO.output(Motor_A_Pin1, GPIO.LOW)
GPIO.output(Motor_A_Pin2, GPIO.LOW)
GPIO.output(Motor_B_Pin1, GPIO.LOW)
GPIO.output(Motor_B_Pin2, GPIO.LOW)
pwm_A_EN.ChangeDutyCycle(0)
pwm_B_EN.ChangeDutyCycle(0)
# 定义红色和绿色的HSV值范围
lower_red = np.array([0, 100, 100])
upper_red = np.array([10, 255, 255])
lower_green = np.array([50, 100, 100])
upper_green = np.array([70, 255, 255])
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
# 将视频帧转换为HSV格式
hsv = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
# 提取红色和绿色区域的二进制掩码
mask_red = cv2.inRange(hsv, lower_red, upper_red)
mask_green = cv2.inRange(hsv, lower_green, upper_green)
# 对掩码进行形态学操作,以去除噪声
kernel = np.ones((5,5), np.uint8)
mask_red = cv2.erode(mask_red, kernel, iterations=2)
mask_red = cv2.dilate(mask_red, kernel, iterations=2)
mask_green = cv2.erode(mask_green, kernel, iterations=2)
mask_green = cv2.dilate(mask_green, kernel, iterations=2)
# 找到红色和绿色区域的轮廓
contours_red, _ = cv2.findContours(mask_red, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
contours_green, _ = cv2.findContours(mask_green, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 如果有红色区域,则小车后退
if len(contours_red) > 0:
backward()
time.sleep(0.5)
stop()
time.sleep(0.5)
# 如果有绿色区域,则小车前进
elif len(contours_green) > 0:
forward()
# 否则小车左转
else:
turn_left()
# 显示视频流
cv2.imshow('frame', frame)
# 按下q键退出程序
if cv2.waitKey(1) == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
需要注意的是,该代码中使用了PWM调速控制小车的速度,可以根据实际情况进行调整。此外,HSV值范围也可以根据实际情况进行调整。
阅读全文
相关推荐
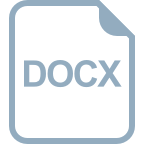
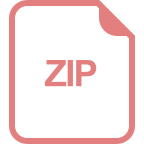
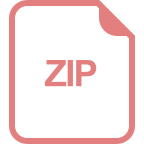















