获取注解的属性
时间: 2024-02-11 15:04:40 浏览: 14
要获取注解的属性,可以使用反射来实现。首先需要获取到被注解的类或方法的Class对象,然后通过该Class对象的getAnnotation方法获取到注解对象,最后调用注解对象的属性方法来获取属性值。
例如,假设我们有一个自定义注解@MyAnnotation,它有一个属性value:
```
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface MyAnnotation {
String value();
}
```
我们可以在一个方法上使用该注解,并给属性value赋值:
```
@MyAnnotation(value = "test")
public void myMethod() {
// ...
}
```
然后在另一个类中,可以使用反射来获取该方法上@MyAnnotation注解的value属性值:
```
Method method = MyClass.class.getMethod("myMethod");
MyAnnotation annotation = method.getAnnotation(MyAnnotation.class);
String value = annotation.value();
```
这样,value变量就会被赋值为"test"。
相关问题
c#反射获取注解属性
C#中可以使用反射来获取带有注解的属性。注解属性可以通过Attribute类的派生类来定义和使用。下面是一个获取注解属性的示例:
假设有以下定义的注解属性:
```csharp
[AttributeUsage(AttributeTargets.Property)]
public class MyAttribute : Attribute
{
public string Name { get; set; }
public MyAttribute(string name)
{
Name = name;
}
}
```
有以下定义的类:
```csharp
public class MyClass
{
[MyAttribute("属性1")]
public string Property1 { get; set; }
[MyAttribute("属性2")]
public string Property2 { get; set; }
}
```
可以使用反射来获取注解属性信息:
```csharp
MyClass myClass = new MyClass();
Type type = myClass.GetType();
PropertyInfo[] properties = type.GetProperties();
foreach (PropertyInfo property in properties)
{
MyAttribute myAttribute = (MyAttribute)Attribute.GetCustomAttribute(property, typeof(MyAttribute));
if (myAttribute != null)
{
Console.WriteLine($"属性名称:{property.Name},注解名称:{myAttribute.Name}");
}
}
```
在上面的示例中,我们使用了Type.GetProperties()方法来获取MyClass类的属性信息,然后使用Attribute.GetCustomAttribute()方法来获取带有MyAttribute注解的属性信息,并输出了属性名称和注解名称。
需要注意的是,Attribute.GetCustomAttribute()方法返回的是一个Attribute类型的对象,需要进行强制类型转换才能获取到注解属性的具体信息。
HttpServletRequest获取注解
HttpServletRequest对象本身并不能直接获取注解,但可以通过反射来获取Servlet类中的注解信息。在给定的例子中,TestServlet类使用了@WebServlet注解,该注解指明了该Servlet的访问路径和初始化顺序等属性。在利用反射获取TestServlet类的注解时,可以使用getAnnotation方法,并传入@WebServlet.class作为参数,以获取@WebServlet注解的相关信息。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [【Servlet-1】HttpServletRequest接口之一](https://blog.csdn.net/qq_42764468/article/details/101373704)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [使用HttpServlet和@WebServlet注解](https://blog.csdn.net/weixin_51992178/article/details/126676450)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
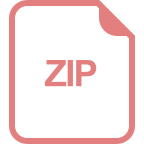
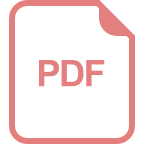
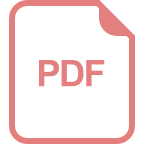












