int[][] map; int n; public MapUtil(int n){ this.n = n; map = new int[n][n]; for(int i=0;i<n;i++){ for(int j=0;j<n;j++){ map[i][j] = -1; } } } public void initMap(){ for(int i=0;i<n;i++){ for(int j=0;j<n;j++){ map[i][j]=(int) (Math.random()*(n-2));///随机生成n-2种图片的ID; } } for(int i=0;i<n;i++){ for(int j=0;j<n;j++){ System.out.print(map[i][j]+" "); } System.out.println(); } }详细解释一下这段代码
时间: 2023-12-09 17:04:57 浏览: 28
这段代码是一个Java类MapUtil的构造函数和两个方法:initMap和printMap。该类的作用是生成一个二维数组map,用于表示一个对对碰游戏的地图。
首先,构造函数MapUtil(int n)接收一个整数参数n,表示地图的大小为n行n列。在构造函数中,通过new int[n][n]创建一个n行n列的二维数组map,然后使用两个for循环将数组map的每个元素初始化为-1,表示该位置没有图片。
接下来,方法initMap()用于随机生成地图中每个位置的图片。在方法中,使用两个for循环遍历数组map的每个元素,然后使用Math.random()方法生成一个0到n-2之间的随机整数作为该位置的图片ID,这样可以保证生成的图片数量为n-2。最后,使用两个for循环再次遍历数组map的每个元素,将该位置的图片ID打印出来,这样可以方便地查看地图的布局情况。
总的来说,这段代码实现了一个对对碰游戏地图的生成和初始化功能,可以作为游戏的基础代码进行扩展和优化。需要注意的是,在实际开发中,还需要实现其他功能,如点击消除、判断是否可以消除等等。
相关问题
public Page(List<T> rows, Integer total, Map<String, Object> eachMap) { this.echoMap = eachMap; this.total = total; this.rows = rows; this.currentPage = (Integer)eachMap.get("currentPage"); this.pageSize = (Integer)eachMap.get("pageSize"); this.totalPage = this.total % this.pageSize == 0 ? this.total / this.pageSize : this.total / this.pageSize + 1; this.firstPage = this.currentPage == 1; this.lastPage = this.currentPage == this.totalPage; this.hasPrev = this.currentPage > 1; this.hasNext = !this.lastPage; if (totalPage < 5) { for (int i = 0; i < totalPage; i++) { this.pageList.add(i + 1); } } else if(totalPage > 5) { for(int i = this.currentPage - 2; i <= this.currentPage + 2; i++) { this.pageList.add(i); } } else { this.pageList.addAll(Arrays.asList(1,2,3,4,5)); } }
这段代码是一个构造函数,它接受一个包含数据行、总数和每个页面的信息的参数,并根据这些信息初始化一个分页对象。
构造函数中的逻辑主要是根据总数和每页大小计算出总页数,并根据当前页码判断是否为第一页或最后一页。然后根据总页数的不同情况,生成页面列表。
如果总页数小于5,将1到总页数的数字依次添加到页面列表中。如果总页数大于5,将当前页码前后2个页码添加到页面列表中。如果总页数等于5,则直接将1到5添加到页面列表中。
最后,将计算得到的各个属性赋值给分页对象的成员变量。
public class ShoppingCar { private double totalPrice; private int totalCount; private Map<Integer, OrderItemBean> itemMap; 为什么map初始化为空 public ShoppingCar() { } public ShoppingCar(double totalPrice, int totalCount, Map<Integer, OrderItemBean> itemMap) { this.totalPrice = 0; this.totalCount = 0; this.itemMap = new HashMap<>(); }
在你提供的代码中,构造函数`ShoppingCar(double totalPrice, int totalCount, Map<Integer, OrderItemBean> itemMap)`中的map初始化为空的原因是,在构造函数中,你重写了初始化map的代码。具体来说,你在构造函数中使用了以下代码:
```
this.itemMap = new HashMap<>();
```
这行代码会创建一个新的空的HashMap对象,并将其赋值给`itemMap`属性。但是,请注意,这行代码会在每次使用该构造函数创建对象时执行,而不是在类的默认构造函数中执行。
因此,如果你在创建`ShoppingCar`对象时使用了带参数的构造函数,并传入了`totalPrice`、`totalCount`和`itemMap`参数,那么map就会被重新初始化为空的HashMap对象。
如果你希望在使用带参数构造函数创建对象时保留原有的map对象,你可以修改构造函数的逻辑,如下所示:
```java
public ShoppingCar(double totalPrice, int totalCount, Map<Integer, OrderItemBean> itemMap) {
this.totalPrice = totalPrice;
this.totalCount = totalCount;
this.itemMap = itemMap;
}
```
这样,当你使用带参数构造函数创建对象时,传入的map对象就会被赋值给`itemMap`属性,而不会重新初始化为空的HashMap对象。
相关推荐
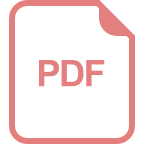
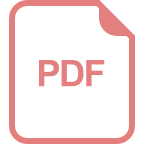
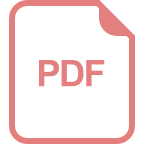












