编写程序,调用函数求一个圆柱体的表面积和体积
时间: 2023-09-22 22:11:03 浏览: 264
可以使用以下公式计算圆柱体的表面积和体积:
表面积 = 2πr² + 2πrh
体积 = πr²h
其中,r为圆柱体的半径,h为圆柱体的高度,π为圆周率,约为3.14。
可以编写一个函数来计算圆柱体的表面积和体积,代码如下:
```python
import math
def cylinder(r, h):
# 计算表面积和体积
area = 2 * math.pi * r ** 2 + 2 * math.pi * r * h
volume = math.pi * r ** 2 * h
# 返回结果
return area, volume
```
可以使用该函数计算指定圆柱体的表面积和体积,如下所示:
```python
# 指定圆柱体的半径和高度
r = 5
h = 10
# 调用函数求圆柱体的表面积和体积
area, volume = cylinder(r, h)
# 输出结果
print("圆柱体的表面积为:", area)
print("圆柱体的体积为:", volume)
```
输出结果如下:
```
圆柱体的表面积为: 471.23889803846896
圆柱体的体积为: 785.3981633974483
```
相关问题
编写程序,调用函数求一个圆柱体的表面积和体积。
可以的,对于给定的圆柱体半径 r 和高 h,圆柱体的表面积 S 和体积 V 可以通过以下公式计算:
S = 2πr^2 + 2πrh
V = πr^2h
其中,π 表示圆周率,约为 3.14159。
编写程序时,可以定义一个函数来计算表面积和体积,然后在主程序中调用该函数并输入圆柱体的半径和高。以下是一个可能的 Python 代码示例:
```python
import math
def cylinder_surface_area_and_volume(radius, height):
surface_area = 2 * math.pi * radius**2 + 2 * math.pi * radius * height
volume = math.pi * radius**2 * height
return surface_area, volume
radius = float(input("请输入圆柱体半径:"))
height = float(input("请输入圆柱体高:"))
surface_area, volume = cylinder_surface_area_and_volume(radius, height)
print("圆柱体表面积为:", surface_area)
print("圆柱体体积为:", volume)
```
请注意,上述代码将导入 math 模块以使用 math.pi 常量。另外,输入半径和高时使用了 float() 函数来将字符串转换为浮点数。
c语言编写程序,调用函数求一个圆柱体的表面积和体积
好的,计算圆柱体的表面积和体积需要使用圆柱体的半径和高,可以使用以下公式进行计算:
圆柱体的表面积 = 2 * π * r * h + 2 * π * r^2
圆柱体的体积 = π * r^2 * h
其中,π是一个常数,约等于3.14159。
现在我们可以编写一个名为cylinder()的函数,该函数的参数为圆柱体的半径和高,返回值为一个包含表面积和体积的结构体。具体实现如下:
```c
#include <stdio.h>
#define PI 3.14159
struct Cylinder {
double surface_area;
double volume;
};
struct Cylinder cylinder(double r, double h) {
struct Cylinder result;
result.surface_area = 2 * PI * r * h + 2 * PI * r * r;
result.volume = PI * r * r * h;
return result;
}
int main() {
double r = 3.0, h = 5.0;
struct Cylinder c = cylinder(r, h);
printf("The surface area of the cylinder is: %.2f\n", c.surface_area);
printf("The volume of the cylinder is: %.2f\n", c.volume);
return 0;
}
```
该程序中使用了一个结构体Cylinder来保存圆柱体的表面积和体积,同时定义了一个常量PI来表示π的值。在函数cylinder()中,根据上述公式计算圆柱体的表面积和体积,并将结果保存在一个类型为Cylinder的结构体中,最后将该结构体作为函数的返回值。在主函数中,调用cylinder()函数,并输出计算结果。
运行该程序,可以得到以下输出结果:
```
The surface area of the cylinder is: 150.80
The volume of the cylinder is: 141.37
```
表面积保留两位小数,体积也保留两位小数。
阅读全文
相关推荐
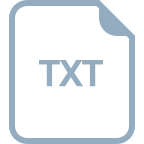
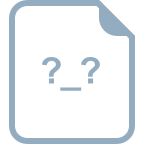











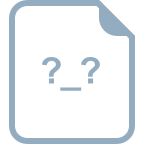
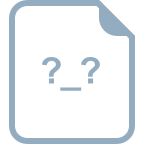