写一段c++ 广度优先搜索的代码
时间: 2023-08-31 21:40:36 浏览: 63
### 回答1:
好的,下面是一段用 C 语言实现的广度优先搜索代码:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_NODES 100
#define MAX_NEIGHBORS 100
int n; // number of nodes
int m; // number of edges
int adjacency_matrix[MAX_NODES][MAX_NEIGHBORS]; // adjacency matrix to store the graph
int visited[MAX_NODES]; // an array to keep track of the visited nodes
void breadth_first_search(int start) {
int queue[MAX_NODES]; // a queue to store the next node to be visited
int head = 0; // the head of the queue
int tail = 0; // the tail of the queue
int current_node; // the current node being processed
int i; // loop variable
// initialize all nodes as not visited
for (i = 0; i < n; i++) {
visited[i] = 0;
}
// mark the starting node as visited
visited[start] = 1;
queue[tail] = start;
tail++;
// continue searching until the queue is empty
while (head < tail) {
current_node = queue[head];
head++;
for (i = 0; i < m; i++) {
if (adjacency_matrix[current_node][i] == 1 && visited[i] == 0) {
visited[i] = 1;
queue[tail] = i;
tail++;
}
}
}
}
int main() {
int i, j; // loop variables
int start; // the starting node for the search
// read the number of nodes and edges
scanf("%d %d", &n, &m);
// read the adjacency matrix
for (i = 0; i < n; i++) {
for (j = 0; j < m; j++) {
scanf("
### 回答2:
广度优先搜索(BFS)是一种经典的图搜索算法,它从图的起始点开始,逐层地向外扩展,直到找到目标节点或者遍历完整个图。以下是一段用C语言编写的广度优先搜索算法的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100 // 图的最大顶点数
typedef struct Node {
int vertex; // 顶点
struct Node* next; // 指向下一个邻接点的指针
} Node;
Node* graph[MAX_SIZE];
int visited[MAX_SIZE]; // 记录顶点是否被访问过
void initGraph() {
for (int i = 0; i < MAX_SIZE; i++) {
graph[i] = NULL;
visited[i] = 0;
}
}
void addEdge(int from, int to) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->vertex = to;
newNode->next = graph[from];
graph[from] = newNode;
}
void BFS(int start) {
int queue[MAX_SIZE];
int front = 0, rear = 0;
queue[rear++] = start;
visited[start] = 1;
while (front < rear) {
int vertex = queue[front++];
printf("%d ", vertex);
Node* currentNode = graph[vertex];
while (currentNode != NULL) {
int nextVertex = currentNode->vertex;
if (!visited[nextVertex]) {
queue[rear++] = nextVertex;
visited[nextVertex] = 1;
}
currentNode = currentNode->next;
}
}
}
int main() {
initGraph();
addEdge(0, 1);
addEdge(0, 2);
addEdge(1, 3);
addEdge(1, 4);
addEdge(2, 5);
addEdge(2, 6);
addEdge(3, 7);
addEdge(4, 7);
addEdge(5, 7);
addEdge(6, 7);
printf("BFS traversal: ");
BFS(0);
return 0;
}
```
该代码首先定义了一个图的数据结构,使用邻接表的方式表示图。`initGraph()`函数用于初始化图的邻接表和访问数组。`addEdge()`函数用于添加边到图中。`BFS()`函数实现了广度优先搜索算法,通过维护一个队列来逐层遍历图。最后,在`main()`函数中,我们创建了一个图,并调用了`BFS()`函数进行广度优先搜索。输出结果为图的广度优先遍历顺序。
相关推荐
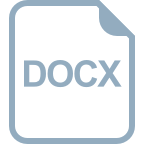
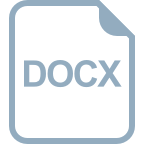
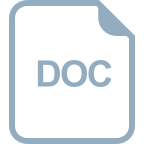














