你能用C++写一段用广度优先遍历的算法解决八数码问题吗
时间: 2024-09-09 12:03:49 浏览: 38
八数码问题是一个经典的搜索问题,通常使用图搜索算法来解决。广度优先搜索(BFS)是一种可以用来解决八数码问题的算法。在BFS中,算法会按照层级顺序遍历状态空间,直到找到目标状态。以下是使用C++实现的八数码问题的广度优先搜索算法的一个简化示例:
```cpp
#include <iostream>
#include <queue>
#include <unordered_map>
#include <vector>
#include <string>
using namespace std;
const vector<pair<int, int>> dirs = {{1, 3}, {0, 2, 4}, {1, 5}, {0, 4}, {1, 3, 5}, {2, 4}, {3, 7}, {4, 6, 8}, {5, 7}};
string move(string state, int zero_pos, int move_pos) {
string new_state = state;
swap(new_state[zero_pos], new_state[move_pos]);
return new_state;
}
bool bfs(string start, string goal) {
if (start == goal) return true;
queue<pair<string, string>> q; // (current state, path)
unordered_map<string, int> depth; // state to depth
q.push({start, ""});
depth[start] = 0;
while (!q.empty()) {
auto [state, path] = q.front();
q.pop();
int zero_pos = state.find('0');
for (int move : dirs[zero_pos]) {
string new_state = move(state, zero_pos, move);
if (depth.find(new_state) == depth.end()) {
if (new_state == goal) {
cout << "Solution found: " << path << " -> " << new_state << endl;
return true;
}
q.push({new_state, new_state});
depth[new_state] = depth[state] + 1;
}
}
}
return false;
}
int main() {
string start = "123456780"; // Initial state of the 3x3 puzzle
string goal = "123456780"; // Goal state, usually in ascending order
if (bfs(start, goal)) {
cout << "The problem can be solved by BFS." << endl;
} else {
cout << "No solution exists using BFS." << endl;
}
return 0;
}
```
这个代码示例中,我们定义了一个`bfs`函数,它接受起始状态和目标状态作为参数。我们使用了一个队列来存储节点和对应的路径信息,并使用一个哈希表来记录每个状态的深度(即从起始状态到当前状态的移动次数)。我们遍历队列中的元素,对于每个状态,我们尝试将0(空白格)移动到所有可能的位置,并将新状态加入到队列中去,如果新状态未被访问过且不是目标状态,就将其深度标记为当前状态深度加一。如果找到目标状态,则返回成功。
请注意,这个代码仅提供了一个算法框架,并没有考虑所有边界情况,例如检查新状态是否有效,或者如何记录路径。实际应用中,还需要添加相应的代码来处理这些情况。
相关推荐
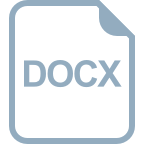
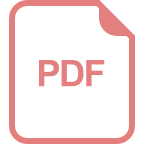
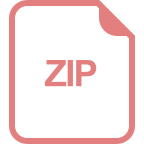














