编写一个C++程序,使用邻接矩阵定义图并实现图的广度优先遍历算法,并在主函数中输出下图从顶点A开始的一个广度优先遍历算法列。 四、思考题 写出上述有向图的一个从顶点A开始的广度优先遍历序列
时间: 2024-12-16 21:21:08 浏览: 11
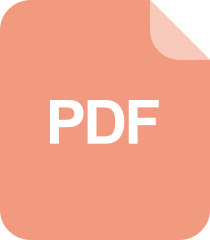
C++实现图的邻接矩阵存储和广度、深度优先遍历实例分析
在C++中,我们可以使用二维数组或者`std::vector<std::vector<int>>`来代表邻接矩阵定义图,这里我将给出一个简单的示例代码来实现图的广度优先遍历(Breadth First Search,BFS)算法。假设我们的有向图是一个4x4的矩阵,其中0表示无边,1表示有边。
```cpp
#include <iostream>
#include <queue>
using namespace std;
#define V 4 // 图的顶点数
// 邻接矩阵表示图
int graph[V][V] = {
{0, 1, 0, 0},
{1, 0, 1, 0},
{0, 1, 0, 1},
{0, 0, 1, 0}
};
// 定义队列结构,用于BFS
struct QueueNode {
int vertex;
QueueNode* next;
};
// 实现队列
QueueNode* createQueueNode(int v) {
QueueNode* newNode = new QueueNode();
newNode->vertex = v;
newNode->next = NULL;
return newNode;
}
void bfs(int start) {
QueueNode* queue = createQueueNode(start);
queue->next = NULL;
cout << "BFS traversal from vertex " << start << ": ";
bool visited[V] = {false}; // 记录已访问节点
while (!queue->next == NULL) {
queue = queue->next; // 出队
cout << queue->vertex << " ";
for (int i = 0; i < V; ++i) {
if (graph[queue->vertex][i] && !visited[i]) {
visited[i] = true;
queue->next = createQueueNode(i); // 入队
break;
}
}
}
}
int main() {
bfs(0); // 从顶点A(索引为0)开始遍历
cout << "\n";
// 思考题:写出从顶点A开始的广度优先遍历序列
阅读全文
相关推荐
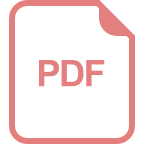
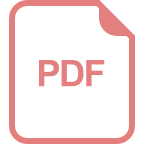






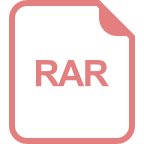
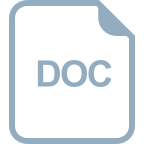







