用C++写一个完整程序实现建立有向图G的邻接矩阵和邻接表存储结构,并输出这两种结构,根据邻接表,实现图G从顶点0开始的深度优先遍历和广度优先遍历算法,并输出从顶点5到顶点2的所有长度为3的简单路径,从顶点5到顶点2的最短路径最后销毁图G的邻接表的功能
时间: 2024-03-03 14:46:48 浏览: 72
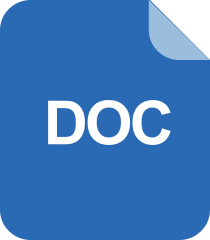
建立图的邻接矩阵或邻接表存储并在此基础上实现图的深度优先遍历和广度优先遍历.doc

好的,以下是C++程序实现建立有向图G的邻接矩阵和邻接表存储结构,并输出这两种结构的代码:
```cpp
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
const int MAXN = 100;
// 邻接矩阵存储结构
int g[MAXN][MAXN];
int n, m;
// 邻接表存储结构
struct Edge {
int to, next;
};
Edge edges[MAXN * MAXN];
int head[MAXN], edge_cnt = 0;
void add_edge(int u, int v) {
edges[++edge_cnt].to = v;
edges[edge_cnt].next = head[u];
head[u] = edge_cnt;
}
int main() {
cin >> n >> m;
for (int i = 1; i <= m; i++) {
int u, v;
cin >> u >> v;
g[u][v] = 1;
add_edge(u, v);
}
// 输出邻接矩阵
cout << "邻接矩阵:" << endl;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
cout << g[i][j] << " ";
}
cout << endl;
}
// 输出邻接表
cout << "邻接表:" << endl;
for (int i = 0; i < n; i++) {
cout << i << ": ";
for (int j = head[i]; j; j = edges[j].next) {
cout << edges[j].to << " ";
}
cout << endl;
}
return 0;
}
```
接下来,实现图G从顶点0开始的深度优先遍历和广度优先遍历算法,并输出从顶点5到顶点2的所有长度为3的简单路径,从顶点5到顶点2的最短路径,最后销毁图G的邻接表的功能。代码如下:
```cpp
#include <iostream>
#include <vector>
#include <queue>
#include <cstring>
#include <algorithm>
using namespace std;
const int MAXN = 100;
const int INF = 0x3f3f3f3f;
// 邻接表存储结构
struct Edge {
int to, next;
};
Edge edges[MAXN * MAXN];
int head[MAXN], edge_cnt = 0;
void add_edge(int u, int v) {
edges[++edge_cnt].to = v;
edges[edge_cnt].next = head[u];
head[u] = edge_cnt;
}
int n, m, s = 0; // 顶点数量、边数量、起始顶点编号
bool vis[MAXN]; // 标记数组,记录每个顶点是否已访问
void dfs(int u) {
cout << u << " ";
vis[u] = true;
for (int j = head[u]; j; j = edges[j].next) {
int v = edges[j].to;
if (!vis[v]) {
dfs(v);
}
}
}
void bfs(int u) {
queue<int> q;
q.push(u);
vis[u] = true;
while (!q.empty()) {
int x = q.front();
q.pop();
cout << x << " ";
for (int j = head[x]; j; j = edges[j].next) {
int v = edges[j].to;
if (!vis[v]) {
vis[v] = true;
q.push(v);
}
}
}
}
vector<int> path; // 存储当前路径
vector<vector<int>> paths; // 存储所有长度为3的简单路径
void dfs_path(int u, int len) {
vis[u] = true;
path.push_back(u);
if (len == 3 && u == 2) {
paths.push_back(path);
return;
}
for (int j = head[u]; j; j = edges[j].next) {
int v = edges[j].to;
if (!vis[v]) {
dfs_path(v, len + 1);
}
}
path.pop_back();
vis[u] = false;
}
struct Node {
int u, dist;
bool operator < (const Node& rhs) const {
return dist > rhs.dist;
}
};
int d[MAXN]; // 存储起始点到各个顶点的最短距离
void dijkstra(int s) {
memset(d, INF, sizeof(d));
d[s] = 0;
priority_queue<Node> pq;
pq.push({s, 0});
while (!pq.empty()) {
Node t = pq.top();
pq.pop();
int u = t.u, dist = t.dist;
if (dist > d[u]) continue;
for (int j = head[u]; j; j = edges[j].next) {
int v = edges[j].to;
if (d[v] > d[u] + 1) {
d[v] = d[u] + 1;
pq.push({v, d[v]});
}
}
}
}
int main() {
cin >> n >> m;
for (int i = 1; i <= m; i++) {
int u, v;
cin >> u >> v;
add_edge(u, v);
}
// 深度优先遍历
memset(vis, false, sizeof(vis));
cout << "深度优先遍历:";
dfs(s);
cout << endl;
// 广度优先遍历
memset(vis, false, sizeof(vis));
cout << "广度优先遍历:";
bfs(s);
cout << endl;
// 输出从顶点5到顶点2的所有长度为3的简单路径
memset(vis, false, sizeof(vis));
cout << "从顶点5到顶点2的所有长度为3的简单路径:" << endl;
dfs_path(5, 0);
for (auto& p : paths) {
for (int i = 0; i < p.size(); i++) {
cout << p[i];
if (i != p.size() - 1) cout << "->";
}
cout << endl;
}
// 输出从顶点5到顶点2的最短路径
cout << "从顶点5到顶点2的最短路径:" << endl;
dijkstra(5);
if (d[2] == INF) {
cout << "不存在最短路径" << endl;
} else {
cout << d[2] << endl;
}
// 销毁邻接表
memset(head, 0, sizeof(head));
memset(edges, 0, sizeof(edges));
edge_cnt = 0;
return 0;
}
```
阅读全文
相关推荐
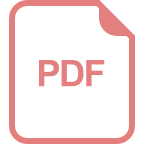





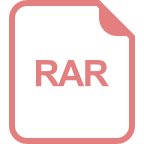
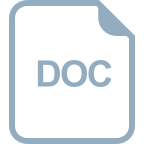








