用c++编写程序设计一个算法将无向图的邻接矩阵转为对应邻接表的算法
时间: 2024-04-15 07:29:59 浏览: 115
当然可以!以下是一个用C++编写的算法,可以将无向图的邻接矩阵转换为对应的邻接表:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 定义无向图类
class Graph {
private:
int numVertices; // 图中顶点的数量
vector<vector<int>> adjMatrix; // 邻接矩阵
public:
// 构造函数
Graph(int n) {
numVertices = n;
// 初始化邻接矩阵
adjMatrix.resize(numVertices, vector<int>(numVertices, 0));
}
// 添加边
void addEdge(int src, int dest) {
adjMatrix[src][dest] = 1;
adjMatrix[dest][src] = 1;
}
// 将邻接矩阵转换为邻接表
vector<vector<int>> convertToAdjList() {
vector<vector<int>> adjList(numVertices);
for (int i = 0; i < numVertices; i++) {
for (int j = 0; j < numVertices; j++) {
if (adjMatrix[i][j] == 1) {
adjList[i].push_back(j);
}
}
}
return adjList;
}
};
int main() {
int numVertices, numEdges;
cout << "Enter the number of vertices: ";
cin >> numVertices;
cout << "Enter the number of edges: ";
cin >> numEdges;
Graph graph(numVertices);
cout << "Enter the edges (source destination):\n";
for (int i = 0; i < numEdges; i++) {
int src, dest;
cin >> src >> dest;
graph.addEdge(src, dest);
}
vector<vector<int>> adjList = graph.convertToAdjList();
cout << "Adjacency List:\n";
for (int i = 0; i < adjList.size(); i++) {
cout << i << " -> ";
for (int j = 0; j < adjList[i].size(); j++) {
cout << adjList[i][j] << " ";
}
cout << endl;
}
return 0;
}
```
这个程序首先创建一个Graph类,它包含一个邻接矩阵和一些函数来添加边和转换为邻接表。在main函数中,我们首先输入图的顶点数量和边数量,然后输入每条边的起点和终点。最后,调用convertToAdjList函数将邻接矩阵转换为邻接表,并打印出来。
希望能帮到你!如有任何疑问,请随时提问。
阅读全文
相关推荐
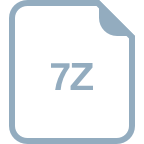
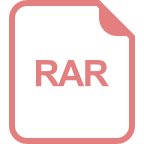
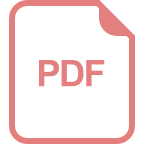















