c++1、以邻接矩阵和邻接表作为存储结构建立一个无向图; 2、按深度优先遍历该无向图,输出遍历序列; 3、按广度优先遍历该无向图,输出遍历序列; 4、采用Dijkstra算法求解某一顶点到其它顶点的最短路径。
时间: 2024-12-13 22:18:58 浏览: 39
1. 在C++中,可以使用邻接矩阵或邻接表这两种数据结构来构建无向图。
- 邻接矩阵:它是一个二维数组,其中行代表源节点,列表示目标节点,元素值表示两个节点之间是否存在边。例如,如果`adj[i][j] = 1`,则表示节点i和节点j之间有边。
- 邻接表:每个节点维护一个链表,链表中包含所有与其相连的节点。对于无向图,通常会使用双向链表,这样可以从任意一头查找到另一头。
2. 深度优先遍历(DFS):
```cpp
void dfs(int node, vector<bool>& visited, vector<vector<int>>& adj) {
visited[node] = true;
cout << node << " ";
for (int neighbor : adj[node]) {
if (!visited[neighbor]) {
dfs(neighbor, visited, adj);
}
}
}
```
遍历序列通过递归调用`dfs`函数完成。
3. 广度优先遍历(BFS):
```cpp
void bfs(vector<int> &queue, int start, vector<bool>& visited, vector<vector<int>>& adj) {
queue.push_back(start);
visited[start] = true;
while (!queue.empty()) {
int current = queue.front();
cout << current << " ";
queue.pop_front();
for (int neighbor : adj[current]) {
if (!visited[neighbor]) {
queue.push_back(neighbor);
visited[neighbor] = true;
}
}
}
}
```
使用队列逐层遍历图。
4. Dijkstra算法求最短路径:
```cpp
struct Node {
int value, dist;
bool operator<(const Node& other) const { return dist < other.dist; }
};
priority_queue<Node> pq;
vector<int> dist(graph.size(), INT_MAX); // 初始化距离为无穷大
dist[vertex] = 0;
for (Node n : graph[vertex]) {
pq.push(n);
}
while (!pq.empty()) {
Node min_node = pq.top(); pq.pop();
int u = min_node.value;
for (auto [v, weight] : graph[u]) {
if (dist[u] + weight < dist[v]) {
dist[v] = dist[u] + weight;
pq.push({v, dist[v]});
}
}
}
```
其中`graph`应为邻接权重矩阵或邻接表形式,这个例子仅给出核心逻辑,实际实现还需要处理边界条件和优化。
阅读全文
相关推荐
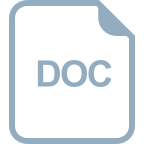
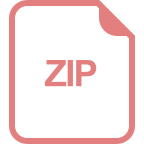
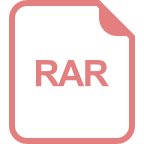















