linux 线程池实现快速排序c++
时间: 2023-11-11 19:02:10 浏览: 104
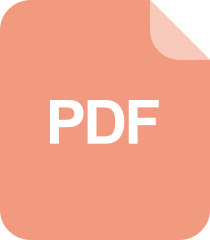
基于线程池的快速排序实现-颜色传感器
以下是一个简单的基于线程池的快速排序实现,使用了 pthread 库和 C++11 的标准库:
```c++
#include <iostream>
#include <vector>
#include <queue>
#include <thread>
#include <mutex>
#include <condition_variable>
std::mutex mtx;
std::condition_variable cv;
std::queue<std::function<void()>> tasks;
std::vector<std::thread> threads;
void sort(std::vector<int>& v, int left, int right) {
if (left < right) {
int pivot = v[left];
int i = left + 1;
int j = right;
while (i <= j) {
if (v[i] <= pivot) {
++i;
} else if (v[j] > pivot) {
--j;
} else {
std::swap(v[i], v[j]);
}
}
std::swap(v[left], v[j]);
sort(v, left, j - 1);
sort(v, j + 1, right);
}
}
void worker() {
while (true) {
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, []{ return !tasks.empty(); });
task = tasks.front();
tasks.pop();
}
task();
}
}
int main() {
const int num_threads = 4;
for (int i = 0; i < num_threads; ++i) {
threads.emplace_back(worker);
}
std::vector<int> v = {4, 6, 2, 8, 5, 7, 1, 9, 3};
int n = v.size();
std::function<void()> task = [&]{
sort(v, 0, n - 1);
};
{
std::unique_lock<std::mutex> lock(mtx);
tasks.emplace(task);
}
cv.notify_one();
for (auto& t : threads) {
t.join();
}
for (int x : v) {
std::cout << x << " ";
}
std::cout << std::endl;
return 0;
}
```
该程序中,我们首先定义了一个线程池,使用了一个条件变量 cv 和一个互斥锁 mtx 来实现线程间的同步和通信。
然后定义了一个 worker 函数,该函数会不断从任务队列中取出任务并执行,直到程序结束。
在主函数中,我们创建了 num_threads 个线程,并将它们的执行函数设置为 worker。然后创建了一个需要排序的数组 v,并定义了一个任务 task,该任务将对数组进行快速排序。
接着,我们将任务加入到任务队列中,并使用条件变量 cv 来通知一个线程可以开始执行任务了。由于我们只有一个任务,因此只需要通知一个线程即可。
最后,我们等待所有线程执行完毕,输出排序后的数组。
注意这里的实现使用了 C++11 的标准库,如果你使用的是 C 语言,可以使用类似的 POSIX 线程库来实现。
阅读全文
相关推荐
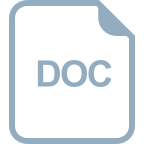
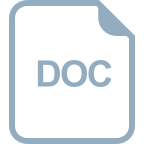
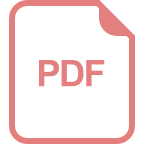
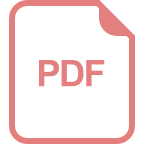
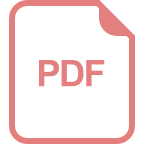
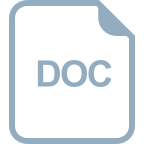
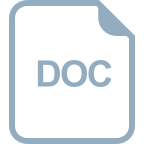
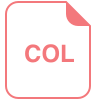
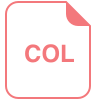
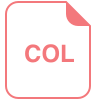
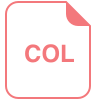
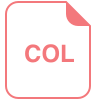
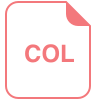
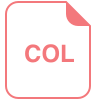
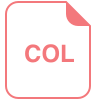
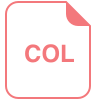
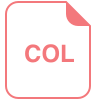