C++定义一个复数类,随机生成一个无序的复数向量(有重复项在顺序的复数向量中,实现区间查找算法,查找出模介于[m1,m2) 的所有元素,按序存于一个子向量中作为返回值。
时间: 2024-05-04 18:15:20 浏览: 182
复数类的定义:
```c++
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
#include <algorithm>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0):real(r), imag(i){}
double getReal() const { return real; }
double getImag() const { return imag; }
double getModule() const { return sqrt(real*real + imag*imag); }
bool operator<(const Complex& c) const { return getModule() < c.getModule(); }
bool operator>=(const Complex& c) const { return getModule() >= c.getModule(); }
friend ostream& operator<<(ostream& os, const Complex& c) {
os << "(" << c.real << ", " << c.imag << ")";
return os;
}
};
```
随机生成一个无序的复数向量:
```c++
vector<Complex> generateRandomComplexVector(int n) {
vector<Complex> vec;
srand(time(nullptr));
for (int i = 0; i < n; i++) {
double real = (double)(rand() % 100) - 50;
double imag = (double)(rand() % 100) - 50;
vec.push_back(Complex(real, imag));
}
return vec;
}
```
区间查找算法:
```c++
vector<Complex> findComplexModuleInRange(const vector<Complex>& vec, double m1, double m2) {
vector<Complex> subVec;
for (auto it = vec.begin(); it != vec.end(); it++) {
if (*it >= Complex(m1) && *it < Complex(m2)) {
subVec.push_back(*it);
}
}
return subVec;
}
```
完整代码:
```c++
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
#include <algorithm>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0):real(r), imag(i){}
double getReal() const { return real; }
double getImag() const { return imag; }
double getModule() const { return sqrt(real*real + imag*imag); }
bool operator<(const Complex& c) const { return getModule() < c.getModule(); }
bool operator>=(const Complex& c) const { return getModule() >= c.getModule(); }
friend ostream& operator<<(ostream& os, const Complex& c) {
os << "(" << c.real << ", " << c.imag << ")";
return os;
}
};
vector<Complex> generateRandomComplexVector(int n) {
vector<Complex> vec;
srand(time(nullptr));
for (int i = 0; i < n; i++) {
double real = (double)(rand() % 100) - 50;
double imag = (double)(rand() % 100) - 50;
vec.push_back(Complex(real, imag));
}
return vec;
}
vector<Complex> findComplexModuleInRange(const vector<Complex>& vec, double m1, double m2) {
vector<Complex> subVec;
for (auto it = vec.begin(); it != vec.end(); it++) {
if (*it >= Complex(m1) && *it < Complex(m2)) {
subVec.push_back(*it);
}
}
return subVec;
}
int main() {
vector<Complex> vec = generateRandomComplexVector(10);
cout << "Original Vector: ";
for (auto i : vec) {
cout << i << " ";
}
cout << endl;
double m1 = 10, m2 = 20;
vector<Complex> subVec = findComplexModuleInRange(vec, m1, m2);
cout << "Sub Vector with Module in [" << m1 << ", " << m2 << "): ";
for (auto i : subVec) {
cout << i << " ";
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐
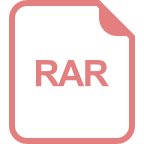
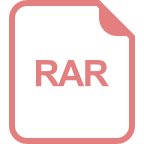
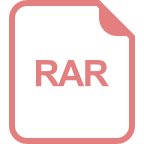
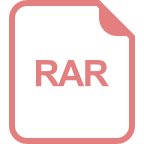
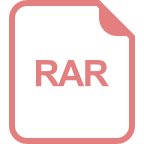
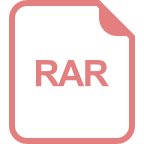
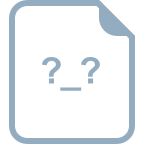
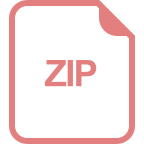
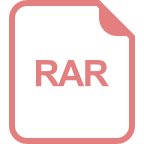
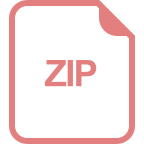
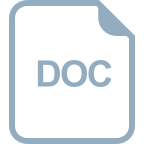