编写字符设备驱动程序,要求能对字符设备执行打开、读、写、IO控制和关闭等基本操作c语言 代码
时间: 2024-01-25 14:02:03 浏览: 20
以下是一个简单的字符设备驱动程序的代码示例:
```c
#include <linux/module.h>
#include <linux/fs.h>
#include <linux/cdev.h>
#include <linux/uaccess.h>
#define DEVICE_NAME "mydevice"
#define BUFFER_SIZE 1024
static int major_number;
static struct cdev mydevice_cdev;
static char buffer[BUFFER_SIZE];
static int mydevice_open(struct inode *inode, struct file *filp)
{
printk(KERN_INFO "mydevice: open\n");
return 0;
}
static int mydevice_release(struct inode *inode, struct file *filp)
{
printk(KERN_INFO "mydevice: release\n");
return 0;
}
static ssize_t mydevice_read(struct file *filp, char __user *buf, size_t count, loff_t *f_pos)
{
printk(KERN_INFO "mydevice: read\n");
if (count > BUFFER_SIZE - *f_pos) {
count = BUFFER_SIZE - *f_pos;
}
if (copy_to_user(buf, buffer + *f_pos, count)) {
return -EFAULT;
}
*f_pos += count;
return count;
}
static ssize_t mydevice_write(struct file *filp, const char __user *buf, size_t count, loff_t *f_pos)
{
printk(KERN_INFO "mydevice: write\n");
if (count > BUFFER_SIZE - *f_pos) {
count = BUFFER_SIZE - *f_pos;
}
if (copy_from_user(buffer + *f_pos, buf, count)) {
return -EFAULT;
}
*f_pos += count;
return count;
}
static long mydevice_ioctl(struct file *filp, unsigned int cmd, unsigned long arg)
{
printk(KERN_INFO "mydevice: ioctl\n");
switch (cmd) {
// TODO: handle ioctl commands
}
return 0;
}
static struct file_operations mydevice_fops = {
.owner = THIS_MODULE,
.open = mydevice_open,
.release = mydevice_release,
.read = mydevice_read,
.write = mydevice_write,
.unlocked_ioctl = mydevice_ioctl,
};
static int __init mydevice_init(void)
{
dev_t dev;
int ret;
ret = alloc_chrdev_region(&dev, 0, 1, DEVICE_NAME);
if (ret < 0) {
printk(KERN_ERR "mydevice: failed to allocate chrdev region\n");
return ret;
}
major_number = MAJOR(dev);
cdev_init(&mydevice_cdev, &mydevice_fops);
mydevice_cdev.owner = THIS_MODULE;
ret = cdev_add(&mydevice_cdev, dev, 1);
if (ret < 0) {
printk(KERN_ERR "mydevice: failed to add cdev\n");
unregister_chrdev_region(MKDEV(major_number, 0), 1);
return ret;
}
printk(KERN_INFO "mydevice: initialized\n");
return 0;
}
static void __exit mydevice_exit(void)
{
cdev_del(&mydevice_cdev);
unregister_chrdev_region(MKDEV(major_number, 0), 1);
printk(KERN_INFO "mydevice: exited\n");
}
module_init(mydevice_init);
module_exit(mydevice_exit);
MODULE_LICENSE("GPL");
```
这个驱动程序注册了一个名为 "mydevice" 的字符设备,支持打开、读、写、IO控制和关闭等基本操作。其中,读和写操作将数据存储在一个静态数组 `buffer` 中,IO控制操作暂时没有实现。
相关推荐
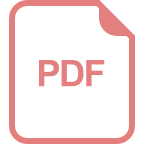
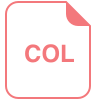
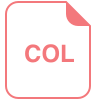
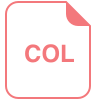
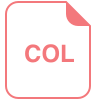
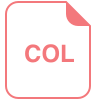









