定义抽象基类Shape,由它派生出五个派生类:Circle(圆形)、Square(正方形)、Rectangle( 长方形)、Trapezoid (梯形)和Triangle (三角形),用虚函数分别计算各种图形的面积,输出它们的面积和。要求用基类指针数组,每一个数组元素指向一个派生类的对象。PI=3.14159f,单精度浮点数计算。 输入格式: 输入在一行中,给出9个大于0的数,用空格分隔,分别代表圆的半径,正方形的边长,矩形的宽和高,梯形的上底、下底和高,三角形的底和高。 输出格式: 输出所有图形的面积和,小数点后保留3位有效数字。
时间: 2024-02-09 21:08:09 浏览: 225
好的,这是一个简单的面向对象程序设计题目,我来帮您解答。
首先,我们需要定义一个抽象基类Shape,其中包含一个虚函数计算面积。代码如下:
```c++
class Shape {
public:
virtual float getArea() = 0;
};
```
然后,我们派生出五个派生类:Circle(圆形)、Square(正方形)、Rectangle(长方形)、Trapezoid(梯形)和Triangle(三角形),分别实现计算面积的虚函数。代码如下:
```c++
class Circle : public Shape {
public:
Circle(float r) : radius(r) {}
float getArea() { return PI * radius * radius; }
private:
float radius;
};
class Square : public Shape {
public:
Square(float s) : side(s) {}
float getArea() { return side * side; }
private:
float side;
};
class Rectangle : public Shape {
public:
Rectangle(float w, float h) : width(w), height(h) {}
float getArea() { return width * height; }
private:
float width, height;
};
class Trapezoid : public Shape {
public:
Trapezoid(float a, float b, float h) : top(a), bottom(b), height(h) {}
float getArea() { return (top + bottom) * height / 2; }
private:
float top, bottom, height;
};
class Triangle : public Shape {
public:
Triangle(float b, float h) : base(b), height(h) {}
float getArea() { return base * height / 2; }
private:
float base, height;
};
```
最后,我们用基类指针数组,每一个数组元素指向一个派生类的对象。代码如下:
```c++
int main() {
float radius, side, width, height, top, bottom, h, base;
cin >> radius >> side >> width >> height >> top >> bottom >> h >> base;
Shape* shapes[5];
shapes[0] = new Circle(radius);
shapes[1] = new Square(side);
shapes[2] = new Rectangle(width, height);
shapes[3] = new Trapezoid(top, bottom, h);
shapes[4] = new Triangle(base, h);
float totalArea = 0;
for (int i = 0; i < 5; i++) {
totalArea += shapes[i]->getArea();
}
cout << fixed << setprecision(3) << totalArea << endl;
return 0;
}
```
完整代码如下:
阅读全文
相关推荐
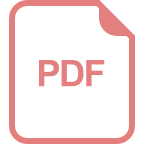















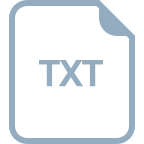