将上述步骤直接写成一个完整完整文件
时间: 2024-03-14 21:46:54 浏览: 31
好的,这里是一个完整的示例文件,它可以在Qt/QML中显示OpenCV获取的摄像头图像。
CameraView.h:
```cpp
#ifndef CAMERAVIEW_H
#define CAMERAVIEW_H
#include <QObject>
#include <QImage>
#include <QTimer>
#include <opencv2/opencv.hpp>
class CameraView : public QObject
{
Q_OBJECT
public:
explicit CameraView(QObject *parent = nullptr);
Q_INVOKABLE void start();
Q_INVOKABLE void stop();
signals:
void imageReady(const QImage &image);
private:
cv::VideoCapture m_capture;
QTimer *m_timer;
};
#endif // CAMERAVIEW_H
```
CameraView.cpp:
```cpp
#include "CameraView.h"
CameraView::CameraView(QObject *parent) : QObject(parent)
{
}
void CameraView::start()
{
m_capture.open(0);
m_timer = new QTimer(this);
connect(m_timer, &QTimer::timeout, this, [this]() {
cv::Mat frame;
m_capture >> frame;
QImage image(frame.data, frame.cols, frame.rows, QImage::Format_RGB888);
emit imageReady(image);
});
m_timer->start(1000 / 30);
}
void CameraView::stop()
{
m_timer->stop();
m_capture.release();
}
```
main.cpp:
```cpp
#include <QGuiApplication>
#include <QQmlApplicationEngine>
#include <QQmlContext>
#include "CameraView.h"
int main(int argc, char *argv[])
{
QCoreApplication::setAttribute(Qt::AA_EnableHighDpiScaling);
QGuiApplication app(argc, argv);
QQmlApplicationEngine engine;
CameraView cameraView;
engine.rootContext()->setContextProperty("cameraView", &cameraView);
const QUrl url(QStringLiteral("qrc:/main.qml"));
QObject::connect(&engine, &QQmlApplicationEngine::objectCreated,
&app, [url](QObject *obj, const QUrl &objUrl) {
if (!obj && url == objUrl)
QCoreApplication::exit(-1);
}, Qt::QueuedConnection);
engine.load(url);
return app.exec();
}
```
main.qml:
```qml
import QtQuick 2.0
Item {
CameraView {
id: camera
}
Image {
id: cameraView
width: 640
height: 480
source: ""
}
Component.onCompleted: {
camera.start()
camera.imageReady.connect(function(image) {
cameraView.source = image
})
}
Component.onDestruction: {
camera.stop()
}
}
```
在这个示例中,我们在 `main.cpp` 中创建了一个 `CameraView` 对象,并将其设置为 `engine` 的 `rootContext` 属性。这样,我们就可以在QML中通过 `cameraView` 访问该对象。在 `main.qml` 中,我们创建了一个 `Image` 元素来显示摄像头图像。我们在 `Component.onCompleted` 信号中连接了 `imageReady` 信号和 `Image` 元素的 `source` 属性,以便在图像就绪时更新它。最后,我们在 `Component.onDestruction` 信号中停止摄像头捕获。
请注意,你需要将 `CameraView.h` 和 `CameraView.cpp` 文件添加到Qt项目中,并在项目文件 `.pro` 中添加以下行:
```pro
INCLUDEPATH += /path/to/opencv/include
LIBS += -L/path/to/opencv/lib -lopencv_core -lopencv_highgui
```
其中 `/path/to/opencv` 应该替换为你的OpenCV安装路径。
相关推荐
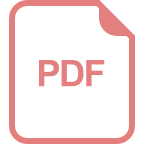
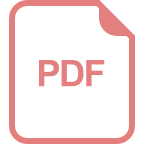
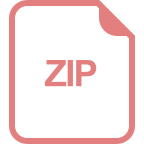














