两个单循环链表的排序连接c语言
时间: 2023-12-04 09:03:23 浏览: 69
以下是两个单循环链表的排序连接C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int val;
struct node* next;
};
typedef struct node Node;
Node* create_node(int val) {
Node* new_node = (Node*) malloc(sizeof(Node));
new_node->val = val;
new_node->next = NULL;
return new_node;
}
Node* create_circular_linked_list(int arr[], int len) {
Node* head = create_node(arr[0]);
Node* tail = head;
for (int i = 1; i < len; i++) {
Node* new_node = create_node(arr[i]);
tail->next = new_node;
tail = new_node;
}
tail->next = head;
return head;
}
void print_circular_linked_list(Node* head) {
Node* cur = head;
do {
printf("%d ", cur->val);
cur = cur->next;
} while (cur != head);
printf("\n");
}
Node* merge_circular_linked_lists(Node* head1, Node* head2) {
if (head1 == NULL) return head2;
if (head2 == NULL) return head1;
Node* cur1 = head1->next;
Node* cur2 = head2->next;
Node* tail = NULL;
if (head1->val < head2->val) {
tail = head1;
cur1 = cur1->next;
} else {
tail = head2;
cur2 = cur2->next;
}
Node* cur = tail;
while (cur1 != head1 && cur2 != head2) {
if (cur1->val < cur2->val) {
cur->next = cur1;
cur1 = cur1->next;
} else {
cur->next = cur2;
cur2 = cur2->next;
}
cur = cur->next;
}
if (cur1 == head1) cur->next = cur2;
else cur->next = cur1;
while (cur->next != tail) cur = cur->next;
cur->next = tail;
return tail;
}
int main() {
int arr1[] = {2, 4, 6};
int len1 = sizeof(arr1) / sizeof(arr1[0]);
Node* head1 = create_circular_linked_list(arr1, len1);
printf("List 1: ");
print_circular_linked_list(head1);
int arr2[] = {1, 3, 5};
int len2 = sizeof(arr2) / sizeof(arr2[0]);
Node* head2 = create_circular_linked_list(arr2, len2);
printf("List 2: ");
print_circular_linked_list(head2);
Node* merged_head = merge_circular_linked_lists(head1, head2);
printf("Merged List: ");
print_circular_linked_list(merged_head);
return 0;
}
```
该代码首先定义了一个节点结构体和一个 create_node 函数,用于创建节点。然后定义了一个 create_circular_linked_list 函数,用于创建循环单链表。接着定义了一个 print_circular_linked_list 函数,用于打印循环单链表。然后定义了一个 merge_circular_linked_lists 函数,用于排序连接两个循环单链表。最后在 main 函数中使用上述函数创建两个循环单链表,排序连接它们,并打印结果。
阅读全文
相关推荐
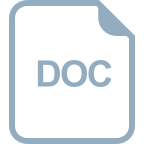
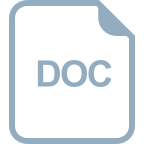
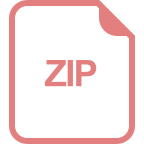
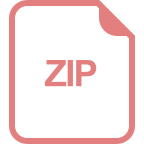
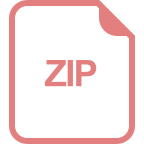
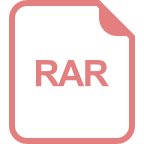
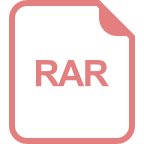
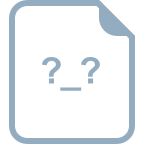
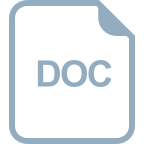
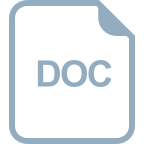
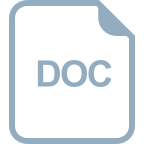
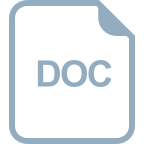
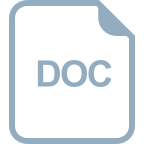
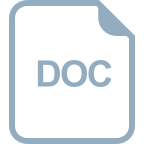
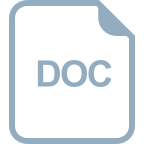
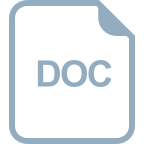
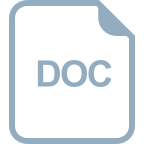