用yolov5s训练完自己的模型后怎么计算fps
时间: 2024-05-04 14:21:52 浏览: 147
要计算使用 YOLOv5s 模型的 FPS(每秒处理帧数),您需要使用以下步骤:
1. 使用 OpenCV 读取视频或摄像头帧。
2. 将每帧输入 YOLOv5s 模型中进行目标检测。
3. 在绘制检测框和标签之前,使用时间戳记录检测开始的时间。
4. 在完成绘制后,使用时间戳记录检测结束的时间。
5. 计算每秒处理帧数(FPS):FPS = 1 / (检测结束时间 - 检测开始时间)。
以下是一个示例代码,可以帮助您计算 FPS:
```
import time
import cv2
import torch
from models.experimental import attempt_load
from utils.general import non_max_suppression
# Load YOLOv5s model
model = attempt_load('models/yolov5s.pt', map_location=torch.device('cpu'))
# Initialize video capture
cap = cv2.VideoCapture(0)
while True:
# Read frame from video
ret, frame = cap.read()
# Perform object detection using YOLOv5s
start_time = time.time()
detections = model(frame)
detections = non_max_suppression(detections, conf_thres=0.5, iou_thres=0.5)
end_time = time.time()
# Draw detection boxes and labels
for det in detections[0]:
x1, y1, x2, y2, conf, cls = det
cv2.rectangle(frame, (int(x1), int(y1)), (int(x2), int(y2)), (0, 255, 0), 2)
cv2.putText(frame, f'{cls}: {conf:.2f}', (int(x1), int(y1) - 10),
cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# Calculate FPS
fps = 1 / (end_time - start_time)
cv2.putText(frame, f'FPS: {fps:.2f}', (10, 30),
cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
# Show frame
cv2.imshow('YOLOv5s', frame)
# Exit on 'q' key
if cv2.waitKey(1) == ord('q'):
break
# Release video capture and close all windows
cap.release()
cv2.destroyAllWindows()
```
上述代码中,我们首先加载了 YOLOv5s 模型,然后使用 `cv2.VideoCapture()` 函数初始化了视频捕获对象。在每个循环迭代中,我们从视频中读取帧并将其输入模型中进行目标检测。检测完成后,我们计算了每秒处理帧数(FPS),并在每个帧上绘制了检测框和标签以及 FPS 值。按下 'q' 键可以退出程序。
阅读全文
相关推荐
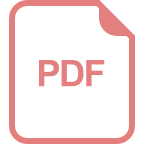
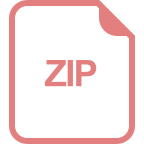
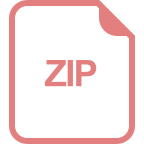








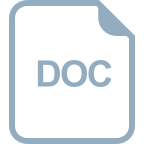
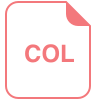
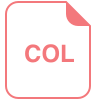




