请用C++写一个线程池
时间: 2023-05-18 10:04:55 浏览: 77
我可以为您编写一个C语言实现的线程池示例: #include<pthread.h>
#define MAX_THREAD 5
/* data structure to pass to the thread functions */
typedef struct thread_data_s {
int id;
} thread_data_t;
/* thread pool context */
typedef struct thread_pool_s {
pthread_t threads[MAX_THREAD];
thread_data_t thread_data[MAX_THREAD];
int thread_count;
} thread_pool_t;
/* thread pool functions */
int thread_pool_init(thread_pool_t *pool);
int thread_pool_add_thread(thread_pool_t *pool, void*(*start_routine)(void*), void *arg);
int thread_pool_wait(thread_pool_t *pool);
void thread_pool_destroy(thread_pool_t *pool);
相关问题
请用C++写一个线程池程序,并注释
//线程池程序:
#include <stdio.h>
#include <pthread.h>
//定义线程池结构体
typedef struct{
void* (*run)(void* args);//函数指针,回调函数
void* args;//回调函数的参数
pthread_t tid;//线程id
int status;//状态
int pool_num;//线程池中线程的数量
}threadpool_t;
//初始化线程池
void threadpool_init(threadpool_t* pool, int pool_num){
pool->pool_num = pool_num;
pool->status = 0;
for(int i=0; i<pool_num; i++){
pthread_create(&(pool->tid), NULL, pool->run, pool->args);//创建线程
}
pool->status = 1;
}
//销毁线程池
void threadpool_destroy(threadpool_t* pool){
pool->status = 0;
for(int i=0; i<pool->pool_num; i++){
pthread_join(pool->tid, NULL);//等待线程结束
}
pool->pool_num = 0;
}
使用c++写一个线程池
下面是一个简单的C++线程池实现,仅供参考:
```c++
#include <iostream>
#include <vector>
#include <queue>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <functional>
#include <future>
class ThreadPool {
public:
ThreadPool(size_t thread_count) : stop(false) {
for (size_t i = 0; i < thread_count; ++i) {
workers.emplace_back(
[this] {
for (;;) {
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(this->queue_mutex);
this->condition.wait(lock,
[this] { return this->stop || !this->tasks.empty(); });
if (this->stop && this->tasks.empty())
return;
task = std::move(this->tasks.front());
this->tasks.pop();
}
task();
}
}
);
}
}
template<class F, class... Args>
auto enqueue(F&& f, Args&&... args) -> std::future<typename std::result_of<F(Args...)>::type> {
using return_type = typename std::result_of<F(Args...)>::type;
auto task = std::make_shared< std::packaged_task<return_type()> >(
std::bind(std::forward<F>(f), std::forward<Args>(args)...)
);
std::future<return_type> res = task->get_future();
{
std::unique_lock<std::mutex> lock(queue_mutex);
// 不允许向已停止的线程池中添加新的任务
if (stop)
throw std::runtime_error("enqueue on stopped ThreadPool");
tasks.emplace([task]() { (*task)(); });
}
condition.notify_one();
return res;
}
~ThreadPool() {
{
std::unique_lock<std::mutex> lock(queue_mutex);
stop = true;
}
condition.notify_all();
for (std::thread& worker : workers)
worker.join();
}
private:
std::vector<std::thread> workers;
std::queue<std::function<void()>> tasks;
std::mutex queue_mutex;
std::condition_variable condition;
bool stop;
};
```
上面的代码中,`ThreadPool`类封装了一个线程池,提供了`enqueue()`方法用于向线程池中添加任务。当调用`enqueue()`方法时,线程池会将任务封装成一个`std::packaged_task`对象,并将其放入任务队列中。工作线程会从任务队列中取出任务并执行,直到线程池被销毁或者调用`stop()`方法停止线程池。
可以使用下面的代码测试线程池的功能:
```c++
#include <chrono>
#include <random>
int main() {
ThreadPool pool(4);
std::vector<std::future<int>> results;
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_int_distribution<> dis(1, 100);
for (int i = 0; i < 8; ++i) {
results.emplace_back(
pool.enqueue([i, dis, &gen] {
std::cout << "task " << i << " start" << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(dis(gen)));
std::cout << "task " << i << " end" << std::endl;
return i * i;
})
);
}
for (auto&& result : results)
std::cout << result.get() << ' ';
std::cout << std::endl;
return 0;
}
```
上面的代码创建了一个包含4个线程的线程池,并向线程池中添加了8个任务。每个任务会随机休眠1~100秒的时间,然后返回任务编号的平方。最后打印所有任务的返回值。
阅读全文
相关推荐
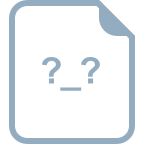
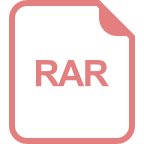









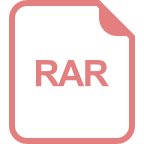
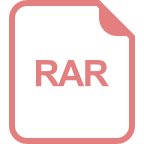