c++ 写一个线程池类
时间: 2023-11-22 18:39:14 浏览: 87
好的,我可以给您提供一个简单的线程池类的实现。具体代码如下:
```
#include <functional>
#include <future>
#include <queue>
#include <thread>
#include <vector>
class ThreadPool {
public:
ThreadPool(size_t threads) : threads_(threads) {
for (size_t i = 0; i < threads_; ++i) {
workers_.emplace_back([this] {
while (true) {
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(mutex_);
condition_.wait(lock, [this] { return !tasks_.empty() || stop_; });
if (stop_ && tasks_.empty()) return;
task = std::move(tasks_.front());
tasks_.pop();
}
task();
}
});
}
}
~ThreadPool() {
{
std::unique_lock<std::mutex> lock(mutex_);
stop_ = true;
}
condition_.notify_all();
for (auto& worker : workers_) {
worker.join();
}
}
template <typename Func, typename... Args>
auto enqueue(Func&& func, Args&&... args)
-> std::future<typename std::result_of<Func(Args...)>::type> {
using return_type = typename std::result_of<Func(Args...)>::type;
auto task = std::make_shared<std::packaged_task<return_type()>>(
std::bind(std::forward<Func>(func), std::forward<Args>(args)...));
std::future<return_type> result = task->get_future();
{
std::unique_lock<std::mutex> lock(mutex_);
tasks_.emplace([task] { (*task)(); });
}
condition_.notify_one();
return result;
}
private:
std::vector<std::thread> workers_;
std::queue<std::function<void()>> tasks_;
std::mutex mutex_;
std::condition_variable condition_;
bool stop_ = false;
};
```
这个线程池类使用C++11标准的语法实现,其中的enqueue()方法可以将任务推入一个队列中,供空闲的线程们去执行。 该类的构造函数会初始化指定数量的线程,并利用lambda函数分别启动这些线程执行任务。 任务队列和线程通信用到的互斥锁和条件变量保证了线程安全。同时,我们也可以在应用程序退出时调用析构函数,等待所有线程执行完毕并退出来保证程序正常结束。
阅读全文
相关推荐
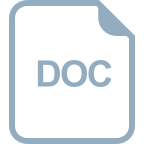
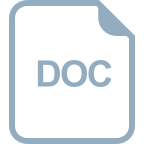
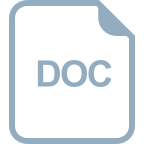












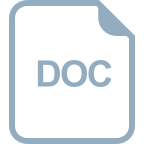
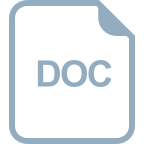
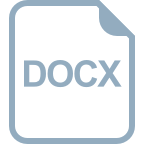
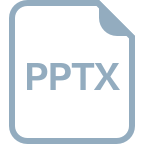