c++写一个线程池类,并附带注释
时间: 2024-04-29 14:26:11 浏览: 112
我可以帮你回答这个问题,以下是一个简单的线程池类的示例代码:
```
#include <thread>
#include <mutex>
#include <condition_variable>
#include <queue>
#include <functional>
class ThreadPool {
public:
ThreadPool(std::size_t poolSize) : stop(false) {
// 创建线程池中的工作线程并启动
for (std::size_t i = 0; i < poolSize; ++i)
workers.emplace_back(
[this] {
while (true) {
std::unique_lock<std::mutex> lock(this->mtx);
this->condition.wait(lock,
[this] { return this->stop || !this->tasks.empty(); });
if (this->stop && this->tasks.empty())
return;
std::function<void()> task(this->tasks.front().release());
this->tasks.pop();
lock.unlock();
task(); // 执行任务
}
}
);
}
// 添加新任务到线程池中
template<class F, class... Args>
auto enqueue(F&& f, Args&&... args)
-> std::future<typename std::result_of<F(Args...)>::type> {
using return_type = typename std::result_of<F(Args...)>::type;
auto task = std::make_shared<std::packaged_task<return_type()>>(
std::bind(std::forward<F>(f), std::forward<Args>(args)...)
);
std::future<return_type> res = task->get_future();
{
std::unique_lock<std::mutex> lock(mtx);
if (stop)
throw std::runtime_error("enqueue on stopped ThreadPool");
tasks.emplace([task]() { (*task)(); });
}
condition.notify_one();
return res;
}
~ThreadPool() {
{
std::unique_lock<std::mutex> lock(mtx);
stop = true;
}
condition.notify_all();
for (std::thread &worker : workers)
worker.join();
}
private:
std::vector<std::thread> workers; // 线程池中的工作线程
std::queue<std::unique_ptr<std::function<void()>>> tasks; // 待执行的任务队列
std::mutex mtx; // 保证对任务队列的访问是线程安全的
std::condition_variable condition; // 任务队列为空时等待的条件变量
bool stop; // 表示线程池是否停止运行
};
```
这个线程池类包含一个待执行任务队列,以及一个固定数量的工作线程,用于处理任务队列中的任务。当线程池初始化时,会创建并启动指定数量的工作线程。当用户需要将新任务添加到线程池中时,可以调用线程池的 enqueue 方法,并将任务的函数对象和参数传递给该方法。enqueue 方法将任务封装成一个 std::packaged_task 对象,并将其添加到待执行任务队列中。当工作线程从队列中获取到一个任务时,会执行该任务。
注释已经在代码中添加了,希望能够对你有所帮助。
阅读全文
相关推荐
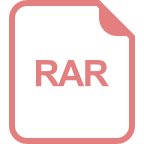


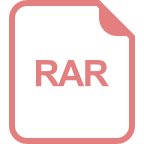
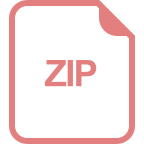
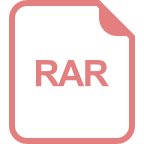
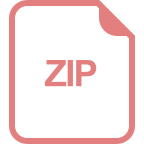
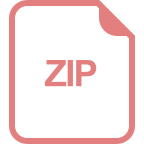
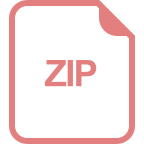
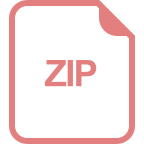
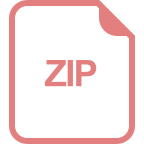
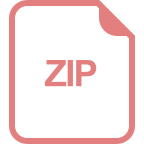