c++写一个简单的线程池,附带备注
时间: 2024-05-15 15:18:11 浏览: 16
好的,这是一个简单的线程池示例代码,附有注释解释每一部分的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define MAX_THREADS 10 // 最大线程数
#define MAX_QUEUE 100 // 最大任务队列长度
typedef struct {
void (*function)(void *); // 任务函数指针
void *data; // 任务数据指针
} task_t;
typedef struct {
pthread_mutex_t lock; // 互斥锁
pthread_cond_t notify; // 条件变量
task_t tasks[MAX_QUEUE]; // 任务队列
int count; // 任务数量
int head; // 任务队列头
int tail; // 任务队列尾
int shutdown; // 线程池关闭标志
pthread_t threads[MAX_THREADS]; // 线程数组
int thread_count; // 线程数量
} threadpool_t;
void *thread_function(void *threadpool);
// 创建线程池
threadpool_t *threadpool_create(int thread_count, int queue_size)
{
threadpool_t *pool;
// 分配空间
if ((pool = (threadpool_t *)malloc(sizeof(threadpool_t))) == NULL)
return NULL;
// 初始化任务队列相关信息
pool->count = 0;
pool->head = 0;
pool->tail = 0;
pool->shutdown = 0;
// 分配空间并初始化互斥锁和条件变量
if (pthread_mutex_init(&(pool->lock), NULL) != 0 || pthread_cond_init(&(pool->notify), NULL) != 0)
{
free(pool);
return NULL;
}
// 创建指定数量的线程
pool->thread_count = 0;
pool->thread_count = thread_count > MAX_THREADS ? MAX_THREADS : thread_count;
for (int i = 0; i < pool->thread_count; i++)
{
if (pthread_create(&(pool->threads[i]), NULL, thread_function, (void *)pool) != 0)
{
threadpool_destroy(pool);
return NULL;
}
}
return pool;
}
// 添加任务到线程池
int threadpool_add(threadpool_t *pool, void (*function)(void *), void *data)
{
int next;
// 对任务队列加锁
pthread_mutex_lock(&(pool->lock));
// 计算下一个任务将加入队列的位置
next = pool->tail + 1;
next = next == MAX_QUEUE ? 0 : next;
// 任务队列已满
if (pool->count == MAX_QUEUE)
return -1;
// 添加任务到队列中,并更新任务相关信息
pool->tasks[pool->tail].function = function;
pool->tasks[pool->tail].data = data;
pool->tail = next;
pool->count++;
// 唤醒线程来执行任务
pthread_cond_signal(&(pool->notify));
// 解锁
pthread_mutex_unlock(&(pool->lock));
return 0;
}
// 销毁线程池
int threadpool_destroy(threadpool_t *pool)
{
int i;
// 线程状态标志设为关闭
pool->shutdown = 1;
// 唤醒所有线程并等待其结束
pthread_cond_broadcast(&(pool->notify));
for (i = 0; i < pool->thread_count; i++)
pthread_join(pool->threads[i], NULL);
// 释放锁相关资源
pthread_mutex_destroy(&(pool->lock));
pthread_cond_destroy(&(pool->notify));
// 释放线程池相关资源
free(pool);
pool = NULL;
return 0;
}
// 线程函数(不断从任务队列中取出任务并执行)
void *thread_function(void *threadpool)
{
threadpool_t *pool = (threadpool_t *)threadpool;
task_t task;
while (1)
{
// 对任务队列加锁
pthread_mutex_lock(&(pool->lock));
// 等待通知或者任务队列有任务
while (pool->count == 0 && !pool->shutdown)
pthread_cond_wait(&(pool->notify), &(pool->lock));
// 退出线程释放相关资源
if (pool->shutdown)
{
pthread_mutex_unlock(&(pool->lock));
pthread_exit(NULL);
}
// 取出任务并更新任务队列相关信息
task.function = pool->tasks[pool->head].function;
task.data = pool->tasks[pool->head].data;
pool->head = (pool->head + 1) == MAX_QUEUE ? 0 : pool->head + 1;
pool->count--;
// 解锁
pthread_mutex_unlock(&(pool->lock));
// 执行任务函数
(*(task.function))(task.data);
}
pthread_exit(NULL);
}
```
该示例代码实现了一个简单的线程池,允许添加任务到线程池执行。它使用互斥锁和条件变量等线程同步机制来保证线程安全。其中,线程函数中不断从任务队列中取出任务并执行,直到线程池关闭。www.z10z.com
相关推荐
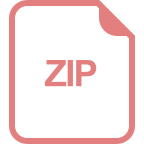
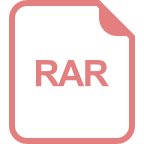














