给定一棵二叉树,设计一个算法,创建含有某一深度上所有节点的链表(比如,若一棵树的深度为 D,则会创建出 D 个链表)。返回一个包含所有深度的链表的数组。 要二叉树的深度指的是这棵树有几层,比如下面的例子深度为4,也就是需要返回1个包含4个链表的数组
时间: 2024-03-26 08:34:11 浏览: 367
好的,这道题目可以使用广度优先搜索(BFS)来解决。具体思路是从根节点开始,进行BFS,每一层的节点都放入一个链表中,最终返回所有链表的数组。
以下是代码实现:
```python
def listOfDepth(tree):
if not tree:
return []
res = []
queue = [tree]
while queue:
size = len(queue)
head = ListNode(0)
cur = head
for i in range(size):
node = queue.pop(0)
cur.next = ListNode(node.val)
cur = cur.next
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
res.append(head.next)
return res
```
其中,ListNode 是一个自定义的链表节点类,可以通过下面的代码创建:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
```
这样,我们就可以通过调用 `listOfDepth` 函数来得到一个包含所有深度的链表的数组。
阅读全文
相关推荐
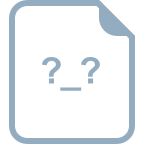















