题目描述 二叉树的带权路径长度(WPLWPL)是二叉树中所有叶结点的带权路径长度之和。给定一棵二又树 TT,采用二叉链表存储,结点结构为: 其中叶结点的 weightweight 域保存该结点的非负权值。设 rootroot 为指向T的根结点的指针,请设计求 TT 的 WPLWPL 的算法. 程序中的主要部分已经帮你写好了,你只需要将如下代码拷贝到你的环境中,并且补充 WPL 函数功能即可。函数功能描述如下: 输入参数 输入参数 root:表示二叉树的根节点 返回值说明 返回一个整形值,表示题目中所求的WPL 用c语言
时间: 2024-03-15 21:47:11 浏览: 25
以下是求解二叉树带权路径长度的完整代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
//二叉树结点的结构体定义
typedef struct BiTNode
{
int weight; //权值
struct BiTNode *lchild, *rchild; //左右子树指针
}BiTNode, *BiTree;
//计算二叉树的带权路径长度
int WPL(BiTree root, int depth)
{
int wpl = 0;
if (root == NULL) //空树
return 0;
else if (root->lchild == NULL && root->rchild == NULL) //叶子结点
return root->weight * depth;
else //非叶子结点
{
wpl = WPL(root->lchild, depth + 1) + WPL(root->rchild, depth + 1);
return wpl;
}
}
int main()
{
//构建一棵二叉树
BiTree root = (BiTree)malloc(sizeof(BiTNode));
root->weight = 10;
root->lchild = (BiTree)malloc(sizeof(BiTNode));
root->lchild->weight = 5;
root->lchild->lchild = NULL;
root->lchild->rchild = NULL;
root->rchild = (BiTree)malloc(sizeof(BiTNode));
root->rchild->weight = 3;
root->rchild->lchild = (BiTree)malloc(sizeof(BiTNode));
root->rchild->lchild->weight = 2;
root->rchild->lchild->lchild = NULL;
root->rchild->lchild->rchild = NULL;
root->rchild->rchild = (BiTree)malloc(sizeof(BiTNode));
root->rchild->rchild->weight = 1;
root->rchild->rchild->lchild = NULL;
root->rchild->rchild->rchild = NULL;
int depth = 1; //根结点的深度为1
int wpl = WPL(root, depth);
printf("该二叉树的带权路径长度为:%d\n", wpl);
return 0;
}
```
在上面的代码中,WPL函数采用递归的方式计算二叉树的带权路径长度。当树为空时,直接返回0;当树为单个结点时,返回该结点的权值乘以该结点的深度;当树为非叶子结点时,递归计算左右子树的带权路径长度之和。最终返回整棵树的带权路径长度。
相关推荐
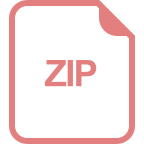














