使用C语言本题要求实现一个函数,统计给定字符串中英文字母、空格或回车、数字字符和其他字符的个数。 函数接口定义: void StringCount( char s[] ); 其中 char s[] 是用户传入的字符串。函数StringCount须在一行内按照 letter = 英文字母个数, blank = 空格或回车个数, digit = 数字字符个数, other = 其他字符个数 的格式输出。
时间: 2024-03-10 22:44:05 浏览: 38
以下是C语言实现的代码:
```c
#include <stdio.h>
#include <ctype.h> // 包含isalpha、isdigit函数
void StringCount(char s[])
{
int letter = 0, blank = 0, digit = 0, other = 0;
// 分别表示英文字母、空格或回车、数字字符和其他字符的个数
for (int i = 0; s[i] != '\0'; i++)
{
if (isalpha(s[i])) // 判断是否为英文字母
letter++;
else if (isspace(s[i])) // 判断是否为空格或回车
blank++;
else if (isdigit(s[i])) // 判断是否为数字字符
digit++;
else // 其他字符
other++;
}
printf("letter = %d, blank = %d, digit = %d, other = %d\n", letter, blank, digit, other);
}
int main()
{
char s[100];
printf("请输入一个字符串:");
gets(s); // gets函数可以读取一行字符串,包括空格和回车
StringCount(s);
return 0;
}
```
注意:gets函数已经被标准C11弃用,应该使用fgets函数代替,具体可参考以下代码:
```c
#include <stdio.h>
#include <ctype.h> // 包含isalpha、isdigit函数
#include <string.h> // 包含strlen函数
void StringCount(char s[])
{
int letter = 0, blank = 0, digit = 0, other = 0;
// 分别表示英文字母、空格或回车、数字字符和其他字符的个数
for (int i = 0; i < strlen(s); i++)
{
if (isalpha(s[i])) // 判断是否为英文字母
letter++;
else if (isspace(s[i])) // 判断是否为空格或回车
blank++;
else if (isdigit(s[i])) // 判断是否为数字字符
digit++;
else // 其他字符
other++;
}
printf("letter = %d, blank = %d, digit = %d, other = %d\n", letter, blank, digit, other);
}
int main()
{
char s[100];
printf("请输入一个字符串:");
fgets(s, 100, stdin); // fgets函数可以读取一行字符串,包括空格和回车
// 将读入的字符串最后的换行符替换成'\0'
if (s[strlen(s) - 1] == '\n')
s[strlen(s) - 1] = '\0';
StringCount(s);
return 0;
}
```
相关推荐
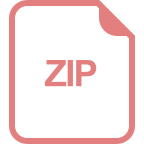
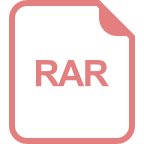
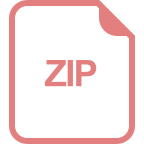
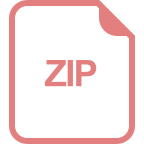
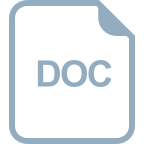
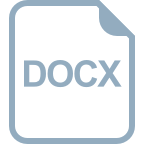
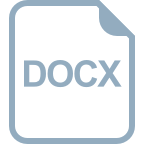
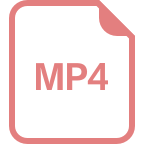
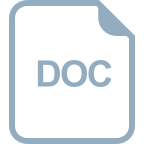