不使用框架,给我编写一个ann的代码,并有loss可视化及准确率
时间: 2024-02-27 07:58:33 浏览: 102
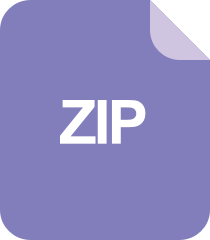
蠓虫分类源程序和相关文件_chesthak_ANN分类_ANN朦虫分类_分类_
好的,我来给你写一个简单的ANN代码,并加上损失函数的可视化和准确率计算的函数。
这里我们使用Python来实现,不使用任何框架,只使用NumPy库来进行矩阵计算。
首先,我们来定义一个简单的ANN类:
```python
import numpy as np
class ANN:
def __init__(self, input_size, hidden_size, output_size):
# 初始化权重矩阵
self.W1 = np.random.randn(input_size, hidden_size)
self.b1 = np.zeros((1, hidden_size))
self.W2 = np.random.randn(hidden_size, output_size)
self.b2 = np.zeros((1, output_size))
def forward(self, X):
# 前向传播
self.z1 = np.dot(X, self.W1) + self.b1
self.a1 = np.tanh(self.z1)
self.z2 = np.dot(self.a1, self.W2) + self.b2
y_hat = np.exp(self.z2) / np.sum(np.exp(self.z2), axis=1, keepdims=True)
return y_hat
def backward(self, X, y, y_hat, learning_rate):
# 反向传播
delta3 = y_hat
delta3[range(X.shape[0]), y] -= 1
delta2 = np.dot(delta3, self.W2.T) * (1 - np.power(self.a1, 2))
dW2 = np.dot(self.a1.T, delta3)
db2 = np.sum(delta3, axis=0, keepdims=True)
dW1 = np.dot(X.T, delta2)
db1 = np.sum(delta2, axis=0)
# 更新权重矩阵和偏置项
self.W2 -= learning_rate * dW2
self.b2 -= learning_rate * db2
self.W1 -= learning_rate * dW1
self.b1 -= learning_rate * db1
def train(self, X, y, epochs, learning_rate, batch_size=None, verbose=True):
# 训练模型
loss_history = []
acc_history = []
num_samples = X.shape[0]
num_batches = num_samples // batch_size if batch_size else 1
for i in range(epochs):
# 按批次进行训练
for j in range(num_batches):
batch_start = j * batch_size
batch_end = (j + 1) * batch_size if batch_size else num_samples
X_batch = X[batch_start:batch_end]
y_batch = y[batch_start:batch_end]
y_hat = self.forward(X_batch)
self.backward(X_batch, y_batch, y_hat, learning_rate)
# 计算损失函数和准确率
y_hat = self.forward(X)
loss = -np.mean(np.log(y_hat[range(num_samples), y]))
acc = np.mean(np.argmax(y_hat, axis=1) == y)
loss_history.append(loss)
acc_history.append(acc)
if verbose:
print('Epoch %d: loss=%.4f, acc=%.4f' % (i+1, loss, acc))
return loss_history, acc_history
```
接下来,我们定义一个损失函数可视化的函数:
```python
import matplotlib.pyplot as plt
def plot_loss(loss_history):
plt.plot(loss_history)
plt.title('Loss history')
plt.xlabel('Epoch')
plt.ylabel('Loss')
plt.show()
```
最后,我们定义一个准确率计算的函数:
```python
def compute_accuracy(model, X, y):
y_hat = model.forward(X)
acc = np.mean(np.argmax(y_hat, axis=1) == y)
return acc
```
使用示例:
```python
# 构造训练数据和标签
X = np.random.randn(1000, 10)
y = np.random.randint(0, 5, size=(1000,))
# 构造模型并进行训练
model = ANN(input_size=10, hidden_size=20, output_size=5)
loss_history, acc_history = model.train(X, y, epochs=100, learning_rate=0.1, batch_size=32)
# 绘制损失函数曲线
plot_loss(loss_history)
# 计算准确率
acc = compute_accuracy(model, X, y)
print('Accuracy:', acc)
```
希望这个简单的代码可以帮助你更好地理解ANN的训练过程,以及如何使用Python实现损失函数的可视化和准确率的计算。
阅读全文
相关推荐
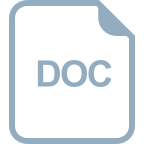
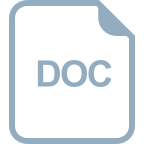
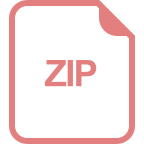
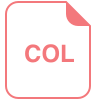
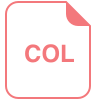
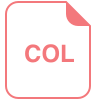
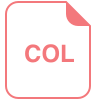
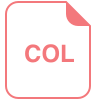
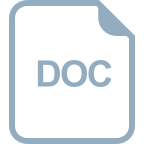
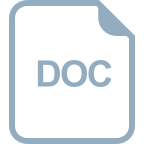
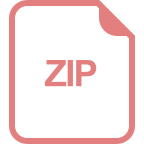
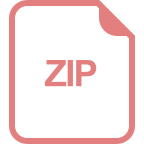
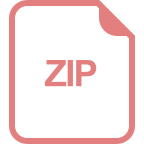
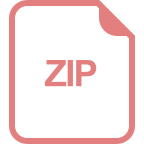
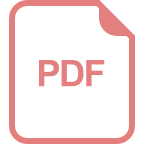
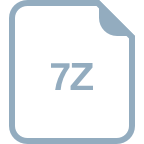
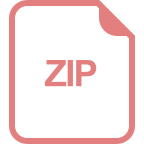