from torch import optim
时间: 2023-09-19 17:07:23 浏览: 119
As an AI language model, I don't have a specific preference for any particular deep learning library as I'm not biased. However, I can provide you with the syntax of the 'optim' module in PyTorch, which is used for defining and updating the parameters of the neural network models. Here is the syntax:
```python
import torch.nn as nn
import torch.optim as optim
# Define the neural network model
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(10, 5)
self.fc2 = nn.Linear(5, 1)
def forward(self, x):
x = nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
# Define the optimizer
net = Net()
optimizer = optim.SGD(net.parameters(), lr=0.01)
# Train the model
for epoch in range(num_epochs):
for i, data in enumerate(train_loader, 0):
inputs, labels = data
optimizer.zero_grad()
outputs = net(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
```
In the above code, we first define a neural network model 'Net' with two fully connected layers. Then, we define an optimizer using the 'optim.SGD' function, which takes the model parameters and the learning rate as inputs. Finally, we train the model by looping through the training data and updating the model parameters using the optimizer.
阅读全文
相关推荐
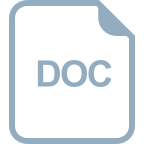
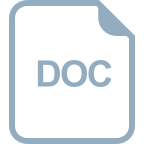
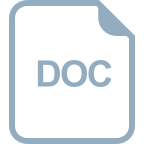















