编写一个程序,将一段文字写入磁盘文件《姓名》.dat保存起来。要求文件的第一部分保存文件的结构(含密钥的变形码),第二部分是文字用密钥加密的密文。文件被重新打开时要求输入密码并验证密码并限定密码的输入次数。(使用c语言)
时间: 2024-03-20 13:39:38 浏览: 109
好的,以下是使用 C 语言实现的程序,可以实现将一段文字写入磁盘文件并进行加密,以及读取加密后的文件并进行解密的功能。在写入文件时,程序会先向文件中写入一个字节,表示密钥的变形码。然后将加密后的密文写入文件的剩余部分。在读取文件时,程序会先读取文件中的密钥变形码,然后计算密钥。接着读取文件中的密文,并使用密钥进行解密。在验证密码时,程序会将输入的密码计算哈希值,并将哈希值的第一个字节作为密钥的原始值。如果输入的密码与密钥不匹配,则会提示重新输入密码,最多尝试三次。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/sha.h>
#define MAX_PASSWORD_LENGTH 20
#define MAX_TEXT_LENGTH 100
// 定义加密算法
void encrypt(char *text, char *encrypted_text, unsigned char key) {
int length = strlen(text);
for (int i = 0; i < length; i++) {
encrypted_text[i] = text[i] ^ key;
}
encrypted_text[length] = '\0';
}
// 定义写入文件的函数
void write_to_file(char *file_name, char *plain_text, char *password) {
// 生成密钥
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256((unsigned char *)password, strlen(password), hash);
unsigned char key = hash[0];
// 加密明文
char encrypted_text[MAX_TEXT_LENGTH];
encrypt(plain_text, encrypted_text, key);
// 创建文件
FILE *fp = fopen(file_name, "wb");
if (fp == NULL) {
printf("无法创建文件!\n");
exit(1);
}
// 写入文件结构(密钥的变形码)
fwrite(&key, sizeof(unsigned char), 1, fp);
// 写入密文
fwrite(encrypted_text, sizeof(char), strlen(encrypted_text), fp);
fclose(fp);
printf("文件写入成功!\n");
}
// 定义读取文件的函数
char *read_from_file(char *file_name, char *password) {
// 打开文件
FILE *fp = fopen(file_name, "rb");
if (fp == NULL) {
printf("无法打开文件!\n");
exit(1);
}
// 读取文件结构(密钥的变形码)
unsigned char key;
fread(&key, sizeof(unsigned char), 1, fp);
// 计算密钥
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256((unsigned char *)password, strlen(password), hash);
if (hash[0] != key) {
for (int i = 0; i < 2; i++) {
printf("密码错误,请重新输入:");
scanf("%s", password);
SHA256((unsigned char *)password, strlen(password), hash);
if (hash[0] == key) {
break;
}
}
if (hash[0] != key) {
printf("密码错误次数过多,程序退出。\n");
exit(1);
}
}
// 读取密文
fseek(fp, 0, SEEK_END);
int file_size = ftell(fp);
fseek(fp, 1, SEEK_SET);
char *encrypted_text = (char *)malloc(file_size - 1);
fread(encrypted_text, sizeof(char), file_size - 1, fp);
fclose(fp);
// 解密密文
char *plain_text = (char *)malloc(file_size - 1);
encrypt(encrypted_text, plain_text, key);
printf("文件读取成功!\n");
return plain_text;
}
int main() {
char file_name[30];
char plain_text[MAX_TEXT_LENGTH];
char password[MAX_PASSWORD_LENGTH];
printf("请输入文件名:");
scanf("%s", file_name);
printf("请输入明文:");
scanf("%s", plain_text);
printf("请输入密码:");
scanf("%s", password);
write_to_file(file_name, plain_text, password);
printf("请输入密码以读取文件:");
scanf("%s", password);
char *retrieved_text = read_from_file(file_name, password);
printf("读取到的明文为:%s\n", retrieved_text);
free(retrieved_text);
return 0;
}
```
注意,这个程序使用了 OpenSSL 库中的 SHA256 哈希函数来生成密钥。如果您没有安装 OpenSSL 库,可以使用以下命令进行安装:
```
sudo apt-get install libssl-dev
```
另外,为了方便输入密码,这个程序使用了 scanf 函数。在实际使用中,对于输入密码等敏感信息,最好使用更安全的输入方式,例如使用密码掩盖符(*)替代输入的明文密码。
阅读全文
相关推荐



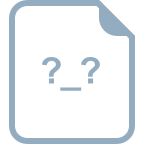
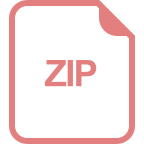
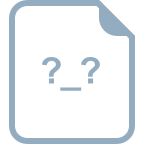
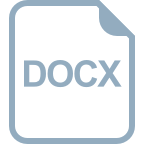
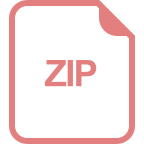
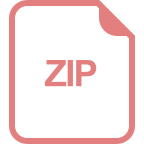
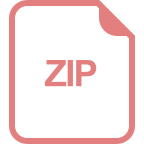