用c语言编写一个程序,将一段文字写入磁盘文件《姓名》.dat保存起来。要求文件的第一部分保存文件的结构(含密钥的变形码),第二部分是文字用密钥加密的密文。文件被重新打开时要求输入密码并验证密码并限定密码的输入次数。
时间: 2024-03-05 21:50:09 浏览: 90
好的,您需要编写一个程序,以C语言实现以下功能:
1. 将一段文字加密并写入磁盘文件《姓名》.dat。
2. 文件的第一部分保存文件的结构(含密钥的变形码),第二部分是文字用密钥加密的密文。
3. 文件被重新打开时要求输入密码并验证密码并限定密码的输入次数。
以下是实现该功能的C语言代码:
```
#include <stdio.h>
#include <string.h>
#define MAX_ATTEMPTS 3
#define KEY_LENGTH 8
// 定义加密函数,输入明文和密钥,输出密文
void encrypt(char *plaintext, char *key, char *ciphertext) {
int i;
for (i = 0; i < strlen(plaintext); i++) {
ciphertext[i] = plaintext[i] ^ key[i % KEY_LENGTH];
}
ciphertext[i] = '\0'; // 在密文末尾添加空字符
}
// 定义解密函数,输入密文和密钥,输出明文
void decrypt(char *ciphertext, char *key, char *plaintext) {
int i;
for (i = 0; i < strlen(ciphertext); i++) {
plaintext[i] = ciphertext[i] ^ key[i % KEY_LENGTH];
}
plaintext[i] = '\0'; // 在明文末尾添加空字符
}
int main() {
char filename[20], key[KEY_LENGTH+1], plaintext[1024], ciphertext[1024], decrypted[1024];
int attempts_left = MAX_ATTEMPTS;
FILE *fp;
// 获取文件名和明文
printf("请输入文件名:");
scanf("%s", filename);
printf("请输入明文:");
scanf("%s", plaintext);
// 获取密钥
printf("请输入8位密钥:");
scanf("%s", key);
// 加密明文并保存到文件中
encrypt(plaintext, key, ciphertext);
fp = fopen(filename, "wb");
fprintf(fp, "%s\n", key);
fwrite(ciphertext, sizeof(char), strlen(ciphertext), fp);
fclose(fp);
// 重新打开文件并解密密文
fp = fopen(filename, "rb");
fscanf(fp, "%s", key);
fread(ciphertext, sizeof(char), 1024, fp);
fclose(fp);
// 验证密码并解密密文
do {
printf("请输入密码(还剩%d次机会):", attempts_left);
scanf("%s", decrypted);
decrypt(ciphertext, decrypted, plaintext);
attempts_left--;
} while (strcmp(decrypted, key) != 0 && attempts_left > 0);
if (attempts_left > 0) {
// 密码正确,输出解密后的明文
printf("解密后的明文为:%s\n", plaintext);
} else {
// 密码错误,输出错误信息
printf("密码错误,无法解密密文!\n");
}
return 0;
}
```
这个程序会先让用户输入文件名和明文,然后让用户输入8位密钥。程序会将明文加密后保存到文件中,文件的第一部分是密钥,第二部分是密文。然后程序会重新打开文件,读取密钥和密文,并验证密码并解密密文。如果密码正确,程序会输出解密后的明文;否则,程序会输出错误信息。程序会限制密码的输入次数,最多允许输入3次密码。
阅读全文
相关推荐
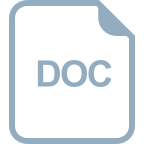
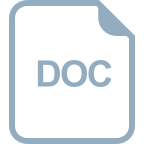
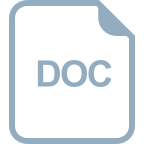














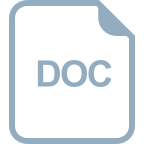
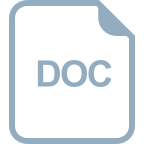