编写一个C语言程序,将一段文字写入磁盘文件《姓名》.dat保存起来。要求文件的 第一部分保存文件的结构(含密钥的变形码),第二部分是文字用密钥加密的密 文。文件被重新打开时要求输入密码并验证密码并限定密码的输入次数。
时间: 2024-03-20 13:38:47 浏览: 80
好的,这是一个比较复杂的程序,需要分步骤来进行编写。我将会提供一个大致的思路以及代码示例,您可以根据需要进行修改和完善。
1. 定义文件结构体
首先,我们需要定义一个结构体来存储文件结构信息,包括文件名、加密密钥、密文长度等。代码示例如下:
```c
typedef struct {
char filename[20]; // 文件名
char key[20]; // 加密密钥
int length; // 密文长度
} FileInfo;
```
2. 编写加密函数
接下来,我们需要编写一个加密函数,将明文转换为密文。这里我们使用简单的凯撒密码进行加密,代码示例如下:
```c
void encrypt(char *plaintext, char *key, char *ciphertext) {
int i, j;
int len = strlen(plaintext);
int keylen = strlen(key);
for (i = 0; i < len; i++) {
j = i % keylen;
ciphertext[i] = (plaintext[i] + key[j] - 'a') % 26 + 'a';
}
ciphertext[len] = '\0';
}
```
3. 编写写入文件函数
接下来,我们需要编写一个函数,将文件结构信息和密文写入磁盘文件。代码示例如下:
```c
void writeFile(FileInfo *fileInfo, char *ciphertext) {
FILE *fp;
fp = fopen(strcat(fileInfo->filename, ".dat"), "wb"); // 以二进制方式打开文件
if (fp == NULL) {
printf("Error: Failed to open file!\n");
return;
}
fwrite(fileInfo, sizeof(FileInfo), 1, fp); // 写入文件结构信息
fwrite(ciphertext, fileInfo->length, 1, fp); // 写入密文
fclose(fp);
printf("File saved successfully!\n");
}
```
4. 编写读取文件函数
接下来,我们需要编写一个函数,从磁盘文件中读取文件结构信息和密文,并进行解密。代码示例如下:
```c
void readFile() {
FileInfo fileInfo;
char *plaintext;
char *ciphertext;
int input_count = 3; // 可输入密码的次数
FILE *fp;
fp = fopen("姓名.dat", "rb"); // 以二进制方式打开文件
if (fp == NULL) {
printf("Error: Failed to open file!\n");
return;
}
fread(&fileInfo, sizeof(FileInfo), 1, fp); // 读取文件结构信息
ciphertext = (char *)malloc(fileInfo.length * sizeof(char));
fread(ciphertext, fileInfo.length, 1, fp); // 读取密文
fclose(fp);
while (input_count > 0) {
char key[20];
printf("Please enter the password: ");
scanf("%s", key);
plaintext = (char *)malloc(fileInfo.length * sizeof(char));
decrypt(ciphertext, key, plaintext); // 解密
if (strcmp(fileInfo.key, key) == 0) {
printf("File opened successfully!\n");
printf("The plaintext is: %s\n", plaintext);
free(plaintext);
break;
} else {
printf("Incorrect password!\n");
input_count--;
free(plaintext);
}
}
if (input_count == 0) {
printf("You have entered the wrong password too many times. The file is locked!\n");
}
free(ciphertext);
}
```
5. 主函数
最后,我们需要编写一个主函数,调用上述函数完成文件的写入和读取操作。代码示例如下:
```c
int main() {
FileInfo fileInfo;
char plaintext[100];
char ciphertext[100];
printf("Please enter the filename: ");
scanf("%s", fileInfo.filename);
printf("Please enter the encryption key: ");
scanf("%s", fileInfo.key);
printf("Please enter the plaintext: ");
getchar();
fgets(plaintext, 100, stdin);
plaintext[strlen(plaintext) - 1] = '\0'; // 去掉换行符
encrypt(plaintext, fileInfo.key, ciphertext);
fileInfo.length = strlen(ciphertext);
writeFile(&fileInfo, ciphertext);
readFile();
return 0;
}
```
以上就是一个简单的文件加密和读取程序的实现。需要注意的是,这里的加密方式非常简单,只是为了演示程序的思路,实际使用中需要使用更加安全的加密方式,例如AES等。
阅读全文
相关推荐
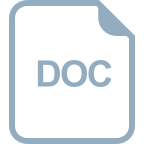
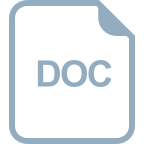
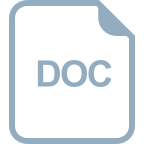














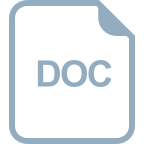
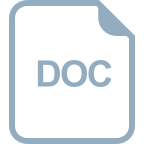