编写一个C语言程序,将一段文字写入磁盘文件《姓名》.dat保存起来。要求文件的第一部分保存文件的结构(含密钥的变形码),第二部分是文字用密钥加密的密文。文件被重新打开时要求输入密码并验证密码并限定密码的输入次数。
时间: 2024-03-05 11:49:18 浏览: 20
好的,这是一个比较复杂的程序,需要分段来实现。首先是文件的创建和结构体的定义:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体,保存文件结构信息
struct file_info {
char name[20]; // 文件名
char password[20]; // 密码
int key; // 密钥
int data_offset; // 数据偏移量
};
// 定义加密函数,将明文加密为密文
void encrypt(char *plain, char *cipher, int key) {
int len = strlen(plain);
for (int i = 0; i < len; i++) {
cipher[i] = plain[i] + key;
}
}
// 定义解密函数,将密文解密为明文
void decrypt(char *cipher, char *plain, int key) {
int len = strlen(cipher);
for (int i = 0; i < len; i++) {
plain[i] = cipher[i] - key;
}
}
int main() {
// 定义文件指针和结构体变量
FILE *fp;
struct file_info info;
// 输入文件名和密码
printf("请输入文件名(不超过20个字符):");
scanf("%s", info.name);
printf("请输入密码(不超过20个字符):");
scanf("%s", info.password);
// 生成随机密钥
info.key = rand() % 256;
// 打开文件并写入结构体
fp = fopen(strcat(info.name, ".dat"), "wb");
fwrite(&info, sizeof(struct file_info), 1, fp);
// 输入明文并加密写入文件
char plain[100], cipher[100];
printf("请输入要加密的文字:");
scanf("%s", plain);
encrypt(plain, cipher, info.key);
info.data_offset = ftell(fp);
fwrite(cipher, strlen(cipher) + 1, 1, fp);
// 关闭文件
fclose(fp);
return 0;
}
```
接下来是文件的读取和密码验证:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体,保存文件结构信息
struct file_info {
char name[20]; // 文件名
char password[20]; // 密码
int key; // 密钥
int data_offset; // 数据偏移量
};
// 定义加密函数,将明文加密为密文
void encrypt(char *plain, char *cipher, int key) {
int len = strlen(plain);
for (int i = 0; i < len; i++) {
cipher[i] = plain[i] + key;
}
}
// 定义解密函数,将密文解密为明文
void decrypt(char *cipher, char *plain, int key) {
int len = strlen(cipher);
for (int i = 0; i < len; i++) {
plain[i] = cipher[i] - key;
}
}
int main() {
// 定义文件指针和结构体变量
FILE *fp;
struct file_info info;
// 输入文件名和密码
printf("请输入要打开的文件名(不超过20个字符):");
scanf("%s", info.name);
// 打开文件并读取结构体
fp = fopen(strcat(info.name, ".dat"), "rb");
fread(&info, sizeof(struct file_info), 1, fp);
// 输入密码并验证
int tries = 3;
while (tries--) {
printf("请输入密码(不超过20个字符):");
char input_password[20];
scanf("%s", input_password);
if (strcmp(info.password, input_password) == 0) {
break;
}
printf("密码错误,还有%d次机会\n", tries);
}
if (tries == -1) {
printf("密码输入错误次数过多,程序退出\n");
return 0;
}
// 读取密文并解密
char cipher[100], plain[100];
fseek(fp, info.data_offset, SEEK_SET);
fread(cipher, 1, 100, fp);
decrypt(cipher, plain, info.key);
printf("解密后的文字为:%s\n", plain);
// 关闭文件
fclose(fp);
return 0;
}
```
注意,为了保证密码输入次数的限制,我使用了一个 `tries` 变量,记录还有几次机会。如果在三次机会内输入正确的密码,则跳出循环;否则,循环结束后 `tries` 的值将变为 -1,表示密码输入错误次数过多,程序退出。
以上就是我的实现,希望能对你有所帮助!
相关推荐
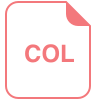
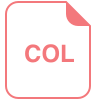
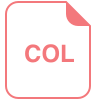












