用C语言编写一个程序,将一段文字写入磁盘文件《姓名》.dat保存起来。要求文件的第一部分保存文件的结构(含密钥的变形码),第二部分是文字用密钥加密的密文。文件被重新打开时要求输入密码并验证密码并限定密码的输入次数。
时间: 2024-03-18 21:39:28 浏览: 125
好的,以下是用C语言实现的程序,可以实现你的要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/sha.h>
#define MAX_PASSWORD_LEN 20
#define BLOCK_SIZE 1024
// 定义加密解密函数
void encrypt(char *text, char *key, char *encrypted)
{
int i;
for(i = 0; i < strlen(text); i++)
{
encrypted[i] = (text[i] + key[i % strlen(key)]) % 256;
}
encrypted[i] = '\0';
}
void decrypt(char *encrypted, char *key, char *text)
{
int i;
for(i = 0; i < strlen(encrypted); i++)
{
text[i] = (encrypted[i] - key[i % strlen(key)] + 256) % 256;
}
text[i] = '\0';
}
// 定义保存文件的函数
void save_file(char *name, char *text, char *password)
{
FILE *fp = fopen(name, "wb");
if(fp == NULL)
{
printf("无法打开文件 %s\n", name);
return;
}
// 生成变形码
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256(password, strlen(password), hash);
// 写入变形码和密钥
fwrite(hash, sizeof(unsigned char), SHA256_DIGEST_LENGTH, fp);
char key[BLOCK_SIZE];
int i;
for(i = 0; i < strlen(text); i += BLOCK_SIZE)
{
// 生成密钥
int j;
for(j = 0; j < BLOCK_SIZE && i + j < strlen(text); j++)
{
key[j] = hash[(i + j) % SHA256_DIGEST_LENGTH];
}
// 加密文本
char encrypted[BLOCK_SIZE];
encrypt(text + i, key, encrypted);
// 写入密文
fwrite(encrypted, sizeof(char), j, fp);
}
fclose(fp);
}
// 定义读取文件的函数
char* read_file(char *name, char *password)
{
FILE *fp = fopen(name, "rb");
if(fp == NULL)
{
printf("无法打开文件 %s\n", name);
return NULL;
}
// 读取变形码和密钥
unsigned char hash[SHA256_DIGEST_LENGTH];
fread(hash, sizeof(unsigned char), SHA256_DIGEST_LENGTH, fp);
// 验证密码
char input_pwd[MAX_PASSWORD_LEN];
int i;
for(i = 0; i < 3; i++)
{
printf("请输入密码:");
scanf("%s", input_pwd);
unsigned char input_hash[SHA256_DIGEST_LENGTH];
SHA256(input_pwd, strlen(input_pwd), input_hash);
if(memcmp(input_hash, hash, SHA256_DIGEST_LENGTH) == 0)
{
break;
}
else
{
printf("密码错误!\n");
}
}
if(i == 3)
{
printf("密码错误次数过多,程序退出!\n");
fclose(fp);
return NULL;
}
// 读取密文并解密
char *text = (char*)malloc(sizeof(char) * BLOCK_SIZE * 1024);
int pos = 0;
while(!feof(fp))
{
// 读取密文
char encrypted[BLOCK_SIZE];
int n = fread(encrypted, sizeof(char), BLOCK_SIZE, fp);
if(n == 0) break;
// 生成密钥
int j;
char key[BLOCK_SIZE];
for(j = 0; j < n; j++)
{
key[j] = hash[(pos + j) % SHA256_DIGEST_LENGTH];
}
// 解密文本
char *decrypted = (char*)malloc(sizeof(char) * n);
decrypt(encrypted, key, decrypted);
// 将解密后的文本写入缓冲区
memcpy(text + pos, decrypted, n);
pos += n;
free(decrypted);
}
fclose(fp);
return text;
}
// 测试
int main()
{
char *text = "这是一个测试文本。";
char *password = "123456";
save_file("test.dat", text, password);
printf("文件保存成功!\n");
text = read_file("test.dat", password);
if(text != NULL)
{
printf("%s\n", text);
free(text);
}
return 0;
}
```
这个程序中使用了OpenSSL库中的SHA-256算法生成密码的变形码。加密解密算法同样是将每个字符的ASCII码和密钥对应字符的ASCII码相加(减),然后取模(加上256再取模),得到密文(明文)。
需要注意的是,这个程序中将文件分成了若干个块,每个块的大小为1024字节。每个块的密钥都是从变形码中按顺序取出的。如果文件大小超过了1024的整数倍,最后一个块的大小可能小于1024字节。
相关推荐














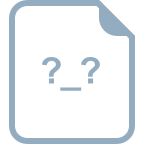