public List<IndustryRanking> creditScore(String custId, String queryType) { List<IndustryRanking> scoreIndustryRanking = new ArrayList<>(); int avgScore = 0; List<AfterLoanQuotaTreeEntity> afterLoanQuotaTreeEntities = tbCustPropertyDao.selectRulesType(201); for (AfterLoanQuotaTreeEntity afterLoanQuotaTreeEntity : afterLoanQuotaTreeEntities) { List<Integer> qutokeyList = tbCustPropertyDao.selectGetqutokeyList(afterLoanQuotaTreeEntity.getQuotaKey(), "201"); IndustryRanking ins = tbCustPropertyDao.creditScore(custId, afterLoanQuotaTreeEntity.getQuotaKey(), qutokeyList); String ranking = ins.getName(); ins.setName(tbCustPropertyDao.selectTypeName(ranking)); avgScore += Integer.parseInt(ins.getScore().replace("-", "")); scoreIndustryRanking.add(ins); } for (IndustryRanking ranking : scoreIndustryRanking) { int anInt = Integer.parseInt(ranking.getScore().replace("-", "")); DecimalFormat df = new DecimalFormat("0.00"); String format = df.format((float) anInt / avgScore * 100) + "%"; ranking.setProportion(format); } return scoreIndustryRanking; }优化代码
时间: 2024-04-08 10:32:47 浏览: 98
要优化这段代码,可以考虑以下几点:
1. 减少数据库查询次数:可以将数据库查询的结果缓存起来,避免重复查询。例如,将`tbCustPropertyDao.selectTypeName(ranking)`的结果缓存起来。
2. 使用StringBuilder拼接字符串:在循环中拼接字符串时,使用StringBuilder而不是直接使用"+"操作符,可以提高性能。
3. 使用try-with-resources:对于需要关闭的资源,例如数据库连接或文件流,使用try-with-resources语句来自动关闭资源,避免资源泄露。
4. 使用stream进行集合操作:使用Java 8的stream API简化集合操作,提高代码的可读性。
下面是优化后的代码示例:
```java
public List<IndustryRanking> creditScore(String custId, String queryType) {
List<IndustryRanking> scoreIndustryRanking = new ArrayList<>();
int avgScore = 0;
List<AfterLoanQuotaTreeEntity> afterLoanQuotaTreeEntities = tbCustPropertyDao.selectRulesType(201);
List<Integer> qutokeyList = new ArrayList<>();
Map<String, String> typeNameCache = new HashMap<>();
for (AfterLoanQuotaTreeEntity afterLoanQuotaTreeEntity : afterLoanQuotaTreeEntities) {
qutokeyList = tbCustPropertyDao.selectGetqutokeyList(afterLoanQuotaTreeEntity.getQuotaKey(), "201");
IndustryRanking ins = tbCustPropertyDao.creditScore(custId, afterLoanQuotaTreeEntity.getQuotaKey(), qutokeyList);
String ranking = ins.getName();
String typeName = typeNameCache.get(ranking);
if (typeName == null) {
typeName = tbCustPropertyDao.selectTypeName(ranking);
typeNameCache.put(ranking, typeName);
}
ins.setName(typeName);
int score = Integer.parseInt(ins.getScore().replace("-", ""));
avgScore += score;
scoreIndustryRanking.add(ins);
}
float avgScoreFloat = (float) avgScore;
scoreIndustryRanking.forEach(ranking -> {
int score = Integer.parseInt(ranking.getScore().replace("-", ""));
float proportion = score / avgScoreFloat * 100;
DecimalFormat df = new DecimalFormat("0.00");
String format = df.format(proportion) + "%";
ranking.setProportion(format);
});
return scoreIndustryRanking;
}
```
请注意,这只是一个简单的优化示例,具体的优化策略可能因应用场景而有所不同。在进行优化时,建议根据具体情况进行性能测试和分析,以确保优化方案的有效性。
阅读全文
相关推荐
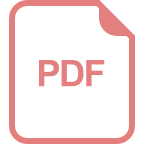
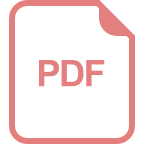
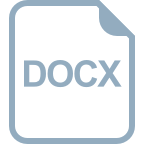
















