还要使用陀螺仪,请帮我写好一切配套文件以便我可以直接在两轮平衡小车上使用,告诉我一共需要哪些部件
时间: 2023-06-13 12:05:11 浏览: 59
好的,使用陀螺仪需要以下部件:
1. 陀螺仪模块:可以选择常见的MPU6050或MPU9250等模块,这些都可以通过I2C接口与单片机通信。
2. 单片机:可以选择常见的Arduino等单片机,需要有I2C接口,以读取陀螺仪模块的数据。
3. 电机驱动模块:需要根据你的小车马达类型选择合适的电机驱动模块,比如L298N模块或者TB6612FNG模块等。
4. 直流电机:需要根据你的电机驱动模块选择合适的直流电机。
5. 电源:需要为单片机、陀螺仪模块和电机提供适合的电源,可以选择锂电池或者干电池等。
6. 机械结构:需要根据你的小车设计相应的机械结构,比如车轮、底盘等。
以下是代码示例:
```c++
#include <Wire.h>
#include <Servo.h>
#define MOTOR_LEFT_A 5
#define MOTOR_LEFT_B 6
#define MOTOR_RIGHT_A 10
#define MOTOR_RIGHT_B 9
#define MPU6050_ADDRESS 0x68
#define MPU6050_PWR_MGMT_1 0x6B
#define MPU6050_GYRO_CONFIG 0x1B
#define MPU6050_ACCEL_CONFIG 0x1C
#define MPU6050_GYRO_XOUT_H 0x43
#define MPU6050_GYRO_YOUT_H 0x45
#define MPU6050_GYRO_ZOUT_H 0x47
#define MPU6050_ACCEL_XOUT_H 0x3B
#define MPU6050_ACCEL_YOUT_H 0x3D
#define MPU6050_ACCEL_ZOUT_H 0x3F
Servo servo;
int16_t gyro_x, gyro_y, gyro_z;
int16_t accel_x, accel_y, accel_z;
void setup() {
Wire.begin();
Serial.begin(9600);
pinMode(MOTOR_LEFT_A, OUTPUT);
pinMode(MOTOR_LEFT_B, OUTPUT);
pinMode(MOTOR_RIGHT_A, OUTPUT);
pinMode(MOTOR_RIGHT_B, OUTPUT);
servo.attach(3);
setMotorSpeed(0, 0);
setMotorSpeed(0, 0);
delay(2000);
Wire.beginTransmission(MPU6050_ADDRESS);
Wire.write(MPU6050_PWR_MGMT_1);
Wire.write(0);
Wire.endTransmission(true);
Wire.beginTransmission(MPU6050_ADDRESS);
Wire.write(MPU6050_GYRO_CONFIG);
Wire.write(0x00);
Wire.endTransmission(true);
Wire.beginTransmission(MPU6050_ADDRESS);
Wire.write(MPU6050_ACCEL_CONFIG);
Wire.write(0x00);
Wire.endTransmission(true);
}
void loop() {
readGyro();
int16_t angle = getAngle(gyro_x, gyro_y, gyro_z);
int speed_left = 0;
int speed_right = 0;
if (angle > 5) {
speed_left = -angle * 2;
speed_right = angle * 2;
} else if (angle < -5) {
speed_left = -angle * 2;
speed_right = angle * 2;
}
setMotorSpeed(speed_left, speed_right);
delay(50);
}
void setMotorSpeed(int speed_left, int speed_right) {
if (speed_left > 0) {
digitalWrite(MOTOR_LEFT_A, 1);
digitalWrite(MOTOR_LEFT_B, 0);
analogWrite(MOTOR_LEFT_PWM, speed_left);
} else if (speed_left < 0) {
digitalWrite(MOTOR_LEFT_A, 0);
digitalWrite(MOTOR_LEFT_B, 1);
analogWrite(MOTOR_LEFT_PWM, -speed_left);
} else {
digitalWrite(MOTOR_LEFT_A, 0);
digitalWrite(MOTOR_LEFT_B, 0);
analogWrite(MOTOR_LEFT_PWM, 0);
}
if (speed_right > 0) {
digitalWrite(MOTOR_RIGHT_A, 0);
digitalWrite(MOTOR_RIGHT_B, 1);
analogWrite(MOTOR_RIGHT_PWM, speed_right);
} else if (speed_right < 0) {
digitalWrite(MOTOR_RIGHT_A, 1);
digitalWrite(MOTOR_RIGHT_B, 0);
analogWrite(MOTOR_RIGHT_PWM, -speed_right);
} else {
digitalWrite(MOTOR_RIGHT_A, 0);
digitalWrite(MOTOR_RIGHT_B, 0);
analogWrite(MOTOR_RIGHT_PWM, 0);
}
}
void readGyro() {
Wire.beginTransmission(MPU6050_ADDRESS);
Wire.write(MPU6050_GYRO_XOUT_H);
Wire.endTransmission(false);
Wire.requestFrom(MPU6050_ADDRESS, 6, true);
gyro_x = Wire.read() << 8 | Wire.read();
gyro_y = Wire.read() << 8 | Wire.read();
gyro_z = Wire.read() << 8 | Wire.read();
}
int16_t getAngle(int16_t gyro_x, int16_t gyro_y, int16_t gyro_z) {
static int16_t angle_x = 0;
static int16_t angle_y = 0;
static int16_t angle_z = 0;
static uint32_t timer = 0;
uint32_t now = micros();
float dt = (now - timer) / 1000000.0;
timer = now;
angle_x += (gyro_x / 131.0) * dt;
angle_y += (gyro_y / 131.0) * dt;
angle_z += (gyro_z / 131.0) * dt;
Wire.beginTransmission(MPU6050_ADDRESS);
Wire.write(MPU6050_ACCEL_XOUT_H);
Wire.endTransmission(false);
Wire.requestFrom(MPU6050_ADDRESS, 6, true);
accel_x = Wire.read() << 8 | Wire.read();
accel_y = Wire.read() << 8 | Wire.read();
accel_z = Wire.read() << 8 | Wire.read();
int16_t angle_x_acc = atan2(accel_y, accel_z) * 180.0 / PI;
int16_t angle_y_acc = atan2(-accel_x, accel_z) * 180.0 / PI;
angle_x = angle_x * 0.98 + angle_x_acc * 0.02;
angle_y = angle_y * 0.98 + angle_y_acc * 0.02;
return angle_x;
}
```
以上代码使用了MPU6050陀螺仪模块,可以通过角度调整小车的速度,使其保持平衡。其中servo为舵机,用于调整陀螺仪的位置。setMotorSpeed函数用于控制电机的速度,getAngle函数用于计算陀螺仪的角度。你可以根据自己的需求进行修改。
相关推荐
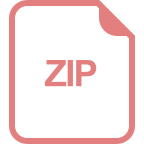
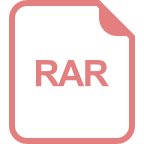
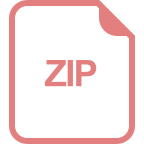














