数据处理中对空值的操作,给我举例一些代码
时间: 2023-03-29 22:04:29 浏览: 88
对空值的操作有很多种,以下是一些常见的代码示例:
1. 删除空值:
```c
for (int i = ; i < n; i++) {
if (data[i] == NULL) {
// 删除空值
for (int j = i; j < n - 1; j++) {
data[j] = data[j + 1];
}
n--;
i--;
}
}
```
2. 填充空值:
```c
for (int i = ; i < n; i++) {
if (data[i] == NULL) {
// 填充空值
data[i] = defaultValue;
}
}
```
3. 跳过空值:
```c
for (int i = ; i < n; i++) {
if (data[i] == NULL) {
// 跳过空值
continue;
}
// 处理非空值
// ...
}
```
以上是一些常见的对空值的操作,具体的实现方式还需要根据具体的需求和数据类型进行调整。
相关问题
如何在Python中准确地判断字符串为空、只含空格或null?请举例说明。
在Python中判断字符串是否为空、仅包含空格或为null值是数据处理中的常见需求,我们可以通过多种方法来实现。以下是一些具体的操作方法和示例代码:
参考资源链接:[Python检查字符串空值与null的三种方法](https://wenku.csdn.net/doc/6412b578be7fbd1778d4344e?spm=1055.2569.3001.10343)
1. **使用字符串长度判断**
首先,我们可以检查字符串的长度是否为0,来判断它是否为空。如果长度为0,则认为字符串为空。
```python
empty_str = ''
print('空字符串' if len(empty_str) == 0 else '非空字符串')
```
2. **使用isspace()判断**
如果字符串不为空,我们还可以检查它是否只包含空白字符。Python的isspace()方法可以用来检测这一点。
```python
space_str = ' '
print('只含空格' if space_str.isspace() else '非只含空格')
```
3. **使用strip()、lstrip()和rstrip()方法**
对于去除字符串两端的空白字符,可以使用strip()方法;要去除左侧空格,使用lstrip();要去除右侧空格,则使用rstrip()。这在处理文本时非常有用。
```python
leading_space = ' leading '
trailing_space = 'trailing '
leading_and_trailing = ' both '
print('左侧空格:', leading_space.lstrip())
print('右侧空格:', trailing_space.rstrip())
print('两端空格:', leading_and_trailing.strip())
```
为了帮助大家更好地理解和应用这些方法,《Python检查字符串空值与null的三种方法》这份资料提供了详尽的示例和解释。它不仅讲解了上述方法,还提供了更多的使用场景和注意事项,非常适合希望深化对字符串处理理解的读者。
掌握这些判断和处理技术对于编写健壮的Python程序至关重要。如果你希望进一步提高在数据处理和文本分析方面的能力,不妨深入学习《Python检查字符串空值与null的三种方法》,它将带领你更加全面地掌握字符串操作的核心知识。
参考资源链接:[Python检查字符串空值与null的三种方法](https://wenku.csdn.net/doc/6412b578be7fbd1778d4344e?spm=1055.2569.3001.10343)
如果文档中含有表格,该如何解析并提取,请给出Python代码示例
如果你的文档中确实包含了表格,并且你想通过Python来提取这些表格的内容,无论是Word还是PDF,都需要借助不同的库。这里以常见的工具举例:
**处理Word文档中的表格**(`python-docx`库):
```python
from docx import Document
from docx.table import Table
def parse_word_table(file_path):
doc = Document(file_path)
tables = doc.tables
parsed_tables = []
for table in tables:
rows = []
for row in table.rows:
cells = [cell.text.strip() for cell in row.cells]
rows.append(cells)
parsed_tables.append(rows)
return parsed_tables
word_file_path = 'your_word_file_with_tables.docx'
parsed_word_tables = parse_word_table(word_file_path)
```
**处理PDF中的表格**(`tabula-py`库,假设表格位于PDF第一页):
```python
import tabula
def parse_pdf_table(file_path):
table = tabula.read_pdf(file_path, pages='1', output_format='dataframe') # 读取第一张表
return table
pdf_file_path = 'your_pdf_file_with_tables.pdf'
parsed_pdf_table = parse_pdf_table(pdf_file_path)
```
请注意,`tabula-py`默认会尝试识别PDF中的表格并转换为Pandas DataFrame,这在大多数情况都能很好地工作。如果表格复杂或者位置不固定,可能需要调整参数或使用其他库如`camelot`。
**合并并处理结果**:
```python
if parsed_word_tables and parsed_pdf_table:
all_tables = parsed_word_tables + parsed_pdf_table
# 对表格数据进行清洗(例如去除空值、统一列名等)
cleaned_tables = [{k: v for k, v in row.items() if v} for row in all_tables]
# 最后,cleaned_tables 就是你想要的表格数据列表
```
阅读全文
相关推荐
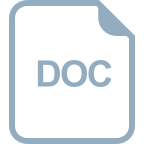
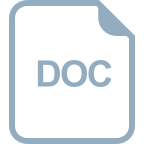
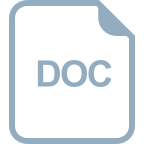
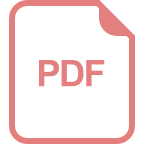
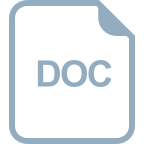
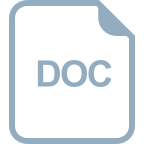
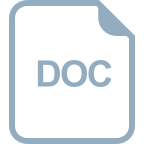
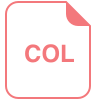
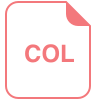
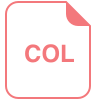
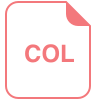
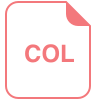
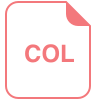
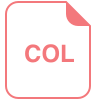
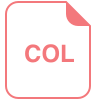
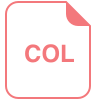
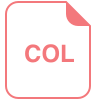