随机产生或键盘输入一组元素,建立一个带头结点的单向链表(无序),遍历单向链表,显示相应元素。
时间: 2024-05-04 17:16:37 浏览: 133
好的,我了解了你的问题。以下是一个C语言的实现,可以实现你的要求:
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct ListNode {
int val; // 元素值
struct ListNode* next; // 下一个节点指针
};
// 创建链表节点
struct ListNode* createNode(int val) {
struct ListNode* new_node = (struct ListNode*) malloc(sizeof(struct ListNode));
new_node->val = val;
new_node->next = NULL;
return new_node;
}
// 插入新节点
void insertNode(struct ListNode* head, int val) {
struct ListNode* new_node = createNode(val);
struct ListNode* curr_node = head;
while (curr_node->next != NULL) {
curr_node = curr_node->next;
}
curr_node->next = new_node;
}
// 遍历链表并显示元素
void traverse(struct ListNode* head) {
struct ListNode* curr_node = head->next;
printf("链表元素:");
while (curr_node != NULL) {
printf("%d ", curr_node->val);
curr_node = curr_node->next;
}
printf("\n");
}
int main() {
// 创建链表头节点
struct ListNode* head = createNode(0);
// 从键盘输入或随机产生一组元素插入链表中
int num;
printf("请输入元素个数:");
scanf("%d", &num);
printf("请输入元素值:");
for (int i = 0; i < num; i++) {
int val;
scanf("%d", &val);
insertNode(head, val);
}
// 遍历链表并显示元素
traverse(head);
// 释放链表空间
struct ListNode* curr_node = head;
while (curr_node != NULL) {
struct ListNode* temp = curr_node;
curr_node = curr_node->next;
free(temp);
}
return 0;
}
```
希望这个代码能够帮助你解决问题!
阅读全文
相关推荐








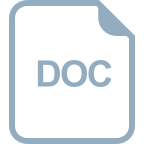
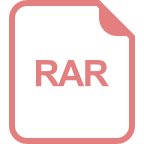


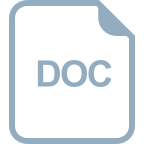



