tensorflow vgg16 python 代码
时间: 2023-07-22 19:12:50 浏览: 107
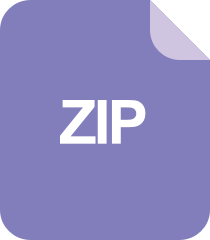
基于tensorflow框架,用训练好的Vgg16模型,实现猫狗图像分类的代码.zip
以下是使用TensorFlow实现VGG16模型的Python代码:
```python
import tensorflow as tf
import numpy as np
import scipy.io
# 加载预训练的VGG16模型
def load_vgg_model(path):
vgg = scipy.io.loadmat(path)
# 获取VGG16模型的参数
vgg_layers = vgg['layers']
def _weights(layer, expected_layer_name):
"""
从VGG模型中获取卷积层的权重和偏置项
"""
W = vgg_layers[0][layer][0][0][2][0][0]
b = vgg_layers[0][layer][0][0][2][0][1]
layer_name = vgg_layers[0][layer][0][0][0][0]
assert layer_name == expected_layer_name
return W,b
def _relu(conv2d_layer):
"""
定义ReLU激活函数
"""
return tf.nn.relu(conv2d_layer)
def _conv2d(prev_layer, layer, layer_name):
"""
定义卷积层
"""
W,b = _weights(layer, layer_name)
W = tf.constant(W)
b = tf.constant(np.reshape(b, (b.size)))
return tf.nn.conv2d(prev_layer, filter=W, strides=[1, 1, 1, 1], padding='SAME') + b
def _conv2d_relu(prev_layer, layer, layer_name):
"""
定义卷积层后接ReLU激活函数
"""
return _relu(_conv2d(prev_layer, layer, layer_name))
def _avgpool(prev_layer):
"""
定义平均池化层
"""
return tf.nn.avg_pool(prev_layer, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME')
# 定义图像输入
graph = {}
graph['input'] = tf.Variable(np.zeros((1, 224, 224, 3)), dtype='float32')
# 定义卷积层
graph['conv1_1'] = _conv2d_relu(graph['input'], 0, 'conv1_1')
graph['conv1_2'] = _conv2d_relu(graph['conv1_1'], 2, 'conv1_2')
graph['avgpool1'] = _avgpool(graph['conv1_2'])
graph['conv2_1'] = _conv2d_relu(graph['avgpool1'], 5, 'conv2_1')
graph['conv2_2'] = _conv2d_relu(graph['conv2_1'], 7, 'conv2_2')
graph['avgpool2'] = _avgpool(graph['conv2_2'])
graph['conv3_1'] = _conv2d_relu(graph['avgpool2'], 10, 'conv3_1')
graph['conv3_2'] = _conv2d_relu(graph['conv3_1'], 12, 'conv3_2')
graph['conv3_3'] = _conv2d_relu(graph['conv3_2'], 14, 'conv3_3')
graph['avgpool3'] = _avgpool(graph['conv3_3'])
graph['conv4_1'] = _conv2d_relu(graph['avgpool3'], 17, 'conv4_1')
graph['conv4_2'] = _conv2d_relu(graph['conv4_1'], 19, 'conv4_2')
graph['conv4_3'] = _conv2d_relu(graph['conv4_2'], 21, 'conv4_3')
graph['avgpool4'] = _avgpool(graph['conv4_3'])
graph['conv5_1'] = _conv2d_relu(graph['avgpool4'], 24, 'conv5_1')
graph['conv5_2'] = _conv2d_relu(graph['conv5_1'], 26, 'conv5_2')
graph['conv5_3'] = _conv2d_relu(graph['conv5_2'], 28, 'conv5_3')
graph['avgpool5'] = _avgpool(graph['conv5_3'])
return graph
```
上述代码定义了一个load_vgg_model函数来加载预训练的VGG16模型,并使用TensorFlow实现了VGG16的网络结构。在这个函数中,我们定义了一系列的卷积层、池化层以及ReLU激活函数,并使用这些层来构建整个VGG16网络结构。最后,我们得到的graph字典包含了整个网络结构的所有层。
阅读全文
相关推荐
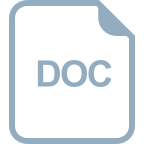
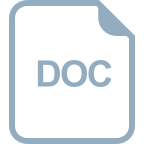
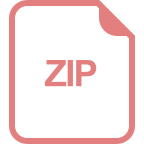
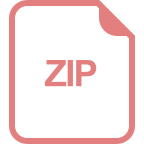
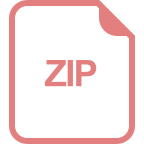


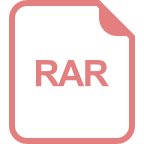
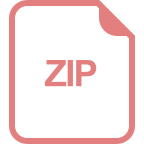
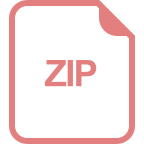
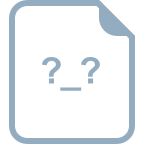






