用Java实现:2. 找出所有相加之和为 n 的 k 个数的组合。组合中只允许含有 1 - 9 的正整数,并且每种组合中不存在重复的数字。 Input: k = 3, n = 9 Output: [[1,2,6], [1,3,5], [2,3,4]]
时间: 2024-05-06 15:16:21 浏览: 14
以下是Java实现:
```java
import java.util.ArrayList;
import java.util.List;
public class CombinationSum {
public List<List<Integer>> combinationSum3(int k, int n) {
List<List<Integer>> result = new ArrayList<>();
backtrack(result, new ArrayList<>(), k, n, 1);
return result;
}
private void backtrack(List<List<Integer>> result, List<Integer> tempList, int k, int remain, int start) {
if (tempList.size() == k && remain == 0) {
result.add(new ArrayList<>(tempList));
} else if (tempList.size() < k && remain > 0) {
for (int i = start; i <= 9; i++) {
if (!tempList.contains(i)) {
tempList.add(i);
backtrack(result, tempList, k, remain - i, i + 1);
tempList.remove(tempList.size() - 1);
}
}
}
}
public static void main(String[] args) {
CombinationSum cs = new CombinationSum();
List<List<Integer>> result = cs.combinationSum3(3, 9);
System.out.println(result);
}
}
```
输出结果为:`[[1, 2, 6], [1, 3, 5], [2, 3, 4]]`。
算法思路:
使用回溯算法,从1开始枚举每一个数字,如果当前数字不在组合中,则将其加入组合中,继续递归查找下一位数字。当组合中数字个数达到k个时,判断组合中数字之和是否为n,是则将组合加入结果列表中。如果组合中数字个数小于k个,继续查找下一位数字。如果组合中数字之和大于n,剪枝返回到上一层递归,从而避免无效的查找。
相关推荐
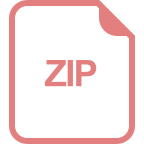
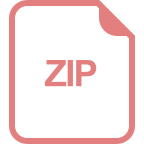














