请用Java写一个二叉树示例代码
时间: 2023-08-31 21:29:01 浏览: 63
### 回答1:
public class BinaryTree {
// Root of Binary Tree
Node root;
// Constructors
BinaryTree(int key)
{
root = new Node(key);
}
BinaryTree()
{
root = null;
}
// 打印节点
public void printInorder(Node node)
{
if (node == null)
return;
/* 使用中序遍历 */
printInorder(node.left);
System.out.print(node.key + " ");
printInorder(node.right);
}
// 中序遍历
public void printInorder() { printInorder(root); }
// 打印前序遍历
public void printPreorder(Node node)
{
if (node == null)
return;
/* 前序遍历 */
System.out.print(node.key + " ");
printPreorder(node.left);
printPreorder(node.right);
}
// 前序遍历
public void printPreorder() { printPreorder(root); }
// 打印后序遍历
public void printPostorder(Node node)
{
if (node == null)
return;
// 后序遍历
printPostorder(node.left);
printPostorder(node.right);
System.out.print(node.key + " ");
}
// 后序遍历
public void printPostorder() { printPostorder(root); }
// 根据给定的键搜索节点
public Node search(Node root, int key)
{
// 根节点为空或者键值与根节点相等
if (root==null || root.key==key)
return root;
// 如果键值小于根节点,则搜索左子树
if (root.key > key)
return search(root.left, key);
// 如果键值大于根节点,则搜索右子树
return search(root.right, key);
}
public static void main(String[] args)
{
BinaryTree tree = new BinaryTree();
/* 创建树 */
tree.root = new Node(1);
tree.root.left = new Node(2);
tree.root.right = new Node(3);
tree.root.left.left = new Node(4);
tree.root.left.right = new Node(5);
System.out.println("中序遍历");
tree.printInorder();
System.out.println("\n前序遍历");
tree.printPreorder();
System.out.println("\n后序遍历");
tree.printPostorder();
}
} // Node类
class Node
{
int key;
Node left, right;
public Node(int item)
{
key = item;
left = right = null;
}
} 答:这是一棵二叉树的示例代码,使用Java编写,可以用中序、前序和后序来遍历树中的节点。
### 回答2:
以下是用Java写的二叉树示例代码:
```java
// 定义二叉树节点类
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int val) {
this.val = val;
this.left = null;
this.right = null;
}
}
// 二叉树类
class BinaryTree {
TreeNode root;
BinaryTree() {
this.root = null;
}
// 插入节点
void insert(int val) {
root = insertNode(root, val);
}
TreeNode insertNode(TreeNode root, int val) {
if (root == null) {
// 如果为空,则创建新节点
root = new TreeNode(val);
return root;
}
// 否则递归地插入左右子树
if (val < root.val)
root.left = insertNode(root.left, val);
else if (val > root.val)
root.right = insertNode(root.right, val);
return root;
}
// 中序遍历
void inorderTraversal() {
inorderTraversal(root);
}
void inorderTraversal(TreeNode root) {
if (root != null) {
inorderTraversal(root.left);
System.out.print(root.val + " ");
inorderTraversal(root.right);
}
}
}
// 测试代码
public class Main {
public static void main(String[] args) {
BinaryTree tree = new BinaryTree();
tree.insert(5);
tree.insert(3);
tree.insert(7);
tree.insert(2);
tree.insert(4);
System.out.println("中序遍历结果:");
tree.inorderTraversal();
}
}
```
该示例代码实现了二叉树的插入节点和中序遍历功能。创建了一个`TreeNode`类来表示二叉树的节点,包含节点的值以及左右子节点的引用。另外,还创建了一个`BinaryTree`类来表示二叉树,包含插入节点和中序遍历两个方法。在`insert()`方法中,使用递归的方式根据节点值的大小插入左右子树。在`inorderTraversal()`方法中,使用递归的方式进行中序遍历,并打印节点的值。
在`main()`方法中,创建一个`BinaryTree`对象,插入了一些节点,并调用`inorderTraversal()`方法进行中序遍历,输出结果。
### 回答3:
下面是用Java编写的一个二叉树的示例代码:
```java
class TreeNode {
int val;
TreeNode left;
TreeNode right;
public TreeNode (int val) {
this.val = val;
this.left = null;
this.right = null;
}
}
public class BinaryTree {
TreeNode root;
public BinaryTree() {
root = null;
}
public void insert(int val) {
root = insertRecursive(root, val);
}
private TreeNode insertRecursive(TreeNode current, int val) {
if (current == null) {
return new TreeNode(val);
}
if (val < current.val) {
current.left = insertRecursive(current.left, val);
} else if (val > current.val) {
current.right = insertRecursive(current.right, val);
}
return current;
}
public boolean search(int val) {
return searchRecursive(root, val);
}
private boolean searchRecursive(TreeNode current, int val) {
if (current == null) {
return false;
}
if (val == current.val) {
return true;
}
if (val < current.val) {
return searchRecursive(current.left, val);
}
return searchRecursive(current.right, val);
}
public void inorderTraversal() {
inorderTraversalRecursive(root);
}
private void inorderTraversalRecursive(TreeNode current) {
if (current != null) {
inorderTraversalRecursive(current.left);
System.out.print(current.val + " ");
inorderTraversalRecursive(current.right);
}
}
public static void main(String[] args) {
BinaryTree tree = new BinaryTree();
tree.insert(5);
tree.insert(3);
tree.insert(7);
tree.insert(2);
tree.insert(4);
tree.insert(6);
tree.insert(8);
System.out.println("Inorder Traversal:");
tree.inorderTraversal();
System.out.println("\nSearching for 6:");
System.out.println(tree.search(6));
System.out.println("Searching for 9:");
System.out.println(tree.search(9));
}
}
```
这个示例代码展示了二叉树的插入、搜索和中序遍历等基本操作。通过 `insert()` 方法可以向二叉树中插入一个新的节点, `search()` 方法用于搜索指定的值是否存在于二叉树中, `inorderTraversal()` 方法用于按中序遍历的顺序输出二叉树的所有节点值。
在 `main()` 方法中,我们创建了一个二叉树,然后插入了一些节点,最后执行了中序遍历和搜索操作,以验证代码的正确性。
相关推荐
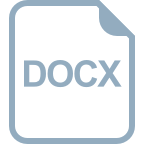
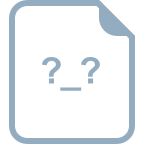














