import torch import torch.nn as nn import torch.nn.functional as F from torch.autograd import Variable class Bottleneck(nn.Module): def init(self, last_planes, in_planes, out_planes, dense_depth, stride, first_layer): super(Bottleneck, self).init() self.out_planes = out_planes self.dense_depth = dense_depth self.conv1 = nn.Conv2d(last_planes, in_planes, kernel_size=1, bias=False) self.bn1 = nn.BatchNorm2d(in_planes) self.conv2 = nn.Conv2d(in_planes, in_planes, kernel_size=3, stride=stride, padding=1, groups=32, bias=False) self.bn2 = nn.BatchNorm2d(in_planes) self.conv3 = nn.Conv2d(in_planes, out_planes+dense_depth, kernel_size=1, bias=False) self.bn3 = nn.BatchNorm2d(out_planes+dense_depth) self.shortcut = nn.Sequential() if first_layer: self.shortcut = nn.Sequential( nn.Conv2d(last_planes, out_planes+dense_depth, kernel_size=1, stride=stride, bias=False), nn.BatchNorm2d(out_planes+dense_depth) ) def forward(self, x): out = F.relu(self.bn1(self.conv1(x))) out = F.relu(self.bn2(self.conv2(out))) out = self.bn3(self.conv3(out)) x = self.shortcut(x) d = self.out_planes out = torch.cat([x[:,:d,:,:]+out[:,:d,:,:], x[:,d:,:,:], out[:,d:,:,:]], 1) out = F.relu(out) return out class DPN(nn.Module): def init(self, cfg): super(DPN, self).init() in_planes, out_planes = cfg['in_planes'], cfg['out_planes'] num_blocks, dense_depth = cfg['num_blocks'], cfg['dense_depth'] self.conv1 = nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1, bias=False) self.bn1 = nn.BatchNorm2d(64) self.last_planes = 64 self.layer1 = self._make_layer(in_planes[0], out_planes[0], num_blocks[0], dense_depth[0], stride=1) self.layer2 = self._make_layer(in_planes[1], out_planes[1], num_blocks[1], dense_depth[1], stride=2) self.layer3 = self._make_layer(in_planes[2], out_planes[2], num_blocks[2], dense_depth[2], stride=2) self.layer4 = self._make_layer(in_planes[3], out_planes[3], num_blocks[3], dense_depth[3], stride=2) self.linear = nn.Linear(out_planes[3]+(num_blocks[3]+1)dense_depth[3], 10) def _make_layer(self, in_planes, out_planes, num_blocks, dense_depth, stride): strides = [stride] + 1 layers = [] for i,stride in (strides): layers.append(Bottleneck(self.last_planes, in_planes, out_planes, dense_depth, stride, i==0)) self.last_planes = out_planes + (i+2) * dense_depth return nn.Sequential(*layers) def forward(self, x): out = F.relu(self.bn1(self.conv1(x))) out = self.layer1(out) out = self.layer2(out) out = self.layer3(out) out = self.layer4(out) out = F.avg_pool2d(out, 4) out = out.view(out.size(0), -1) out = self.linear(out) return out def DPN92(): cfg = { 'in_planes': (96,192,384,768), 'out_planes': (256,512,1024,2048), 'num_blocks': (3,4,20,3), 'dense_depth': (16,32,24,128) } return DPN(cfg)基于这个程序利用pytorch框架修改成对摄像头采集的图像检测与分类输出坐标、大小和种类
时间: 2023-06-10 15:06:35 浏览: 38
这个程序是一个基于DPN网络架构的图像分类模型,需要进行一定的修改才能用于目标检测任务。以下是一些可能需要的修改建议:
1. 修改网络输出层:原网络输出的是一个分类结果,需要修改为输出目标的坐标、大小和种类。可以考虑使用目标检测常用的输出形式,如YOLO等。
2. 加入目标框回归模块:目标检测需要进行目标框回归,可以在网络结构中加入对应的模块。
3. 修改网络输入:原网络输入为3通道的图像,需要将其修改为适合目标检测的形式,如加入多尺度输入等。
4. 加入非极大值抑制(NMS):在输出目标框后需要进行NMS操作,去除重复的框。可以在程序中加入对应的模块。
需要注意的是,以上只是一些基本的修改建议,具体的修改需根据实际任务需求进行选择和调整。
相关问题
AttributeError: module 'torch.nn.modules' has no attribute 'resnet50'
这个错误通常是由于PyTorch版本问题引起的。在较旧的版本中,`resnet50`是在`torchvision.models`中定义的,而在较新的版本中,它被移动到了`torchvision.models.resnet`中。因此,如果您使用的是较旧的版本,请使用以下代码:
```python
import torchvision.models as models
resnet50 = models.resnet50(pretrained=True)
```
如果您使用的是较新的版本,请使用以下代码:
```python
import torch.nn as nn
import torch.utils.model_zoo as model_zoo
model_urls = {
'resnet18': 'https://download.pytorch.org/models/resnet18-5c106cde.pth',
'resnet34': 'https://download.pytorch.org/models/resnet34-333f7ec4.pth',
'resnet50': 'https://download.pytorch.org/models/resnet50-19c8e357.pth',
'resnet101': 'https://download.pytorch.org/models/resnet101-5d3b4d8f.pth',
'resnet152': 'https://download.pytorch.org/models/resnet152-b121ed2d.pth',
}
class ResNet(nn.Module):
def __init__(self, block, layers, num_classes=1000):
self.inplanes = 64
super(ResNet, self).__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=7, stride=2, padding=3,
bias=False)
self.bn1 = nn.BatchNorm2d(64)
self.relu = nn.ReLU(inplace=True)
self.maxpool = nn.MaxPool2d(kernel_size=3, stride=2, padding=1)
self.layer1 = self._make_layer(block, 64, layers[0])
self.layer2 = self._make_layer(block, 128, layers[1], stride=2)
self.layer3 = self._make_layer(block, 256, layers[2], stride=2)
self.layer4 = self._make_layer(block, 512, layers[3], stride=2)
self.avgpool = nn.AdaptiveAvgPool2d((1, 1))
self.fc = nn.Linear(512 * block.expansion, num_classes)
for m in self.modules():
if isinstance(m, nn.Conv2d):
nn.init.kaiming_normal_(m.weight, mode='fan_out', nonlinearity='relu')
elif isinstance(m, (nn.BatchNorm2d, nn.GroupNorm)):
nn.init.constant_(m.weight, 1)
nn.init.constant_(m.bias, 0)
for m in self.modules():
if isinstance(m, Bottleneck):
nn.init.constant_(m.bn3.weight, 0)
def _make_layer(self, block, planes, blocks, stride=1):
downsample = None
if stride != 1 or self.inplanes != planes * block.expansion:
downsample = nn.Sequential(
nn.Conv2d(self.inplanes, planes * block.expansion,
kernel_size=1, stride=stride, bias=False),
nn.BatchNorm2d(planes * block.expansion),
)
layers = []
layers.append(block(self.inplanes, planes, stride, downsample))
self.inplanes = planes * block.expansion
for _ in range(1, blocks):
layers.append(block(self.inplanes, planes))
return nn.Sequential(*layers)
def forward(self, x):
x = self.conv1(x)
x = self.bn1(x)
x = self.relu(x)
x = self.maxpool(x)
x = self.layer1(x)
x = self.layer2(x)
x = self.layer3(x)
x = self.layer4(x)
x = self.avgpool(x)
x = x.view(x.size(0), -1)
x = self.fc(x)
return x
def resnet50(pretrained=False, **kwargs):
model = ResNet(Bottleneck, [3, 4, 6, 3], **kwargs)
if pretrained:
state_dict = model_zoo.load_url(model_urls['resnet50'])
model.load_state_dict(state_dict)
return model
resnet50 = resnet50(pretrained=True)
```
TORCH.UTILS.BOTTLENECK介绍
`torch.utils.bottleneck`是PyTorch中的一个性能优化工具,旨在帮助用户找到代码中的瓶颈并提高代码的运行速度。它提供了一组函数和类,可以在Python解释器中运行代码,并提供有关函数调用时间和内存分配情况的详细信息。对于大型数据集和复杂模型,使用该工具可以显著减少训练和推理时间。使用`bottleneck`时,用户需要将代码包装在一个`with torch.autograd.profiler.profile():`语句块中,以便记录函数调用时间和内存使用情况。同时,`bottleneck`还提供了一些其他的工具,如`optimize_for_inference`函数,可以优化模型以便在推理过程中更快地运行。
相关推荐
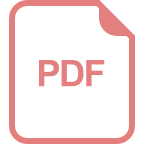
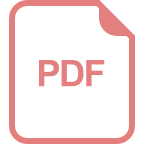
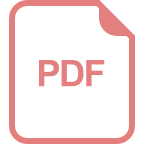










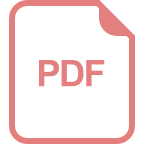
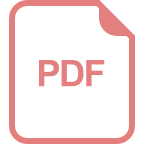
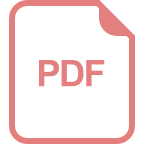
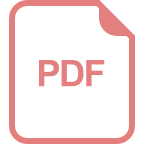