c++ http 图片上传
时间: 2023-10-10 08:05:07 浏览: 43
要实现图片上传,需要使用HTTP协议中的POST方法,将图片以二进制流的方式提交到服务器。以下是一个使用C++实现的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <curl/curl.h>
using namespace std;
static size_t write_callback(char* ptr, size_t size, size_t nmemb, void* userdata)
{
return size * nmemb;
}
int main()
{
CURL* curl = curl_easy_init();
if (!curl)
{
cerr << "Failed to initialize curl" << endl;
return 1;
}
string url = "http://example.com/upload";
string file_path = "image.jpg";
ifstream file(file_path, ios::binary | ios::ate);
if (!file.is_open())
{
cerr << "Failed to open file" << endl;
return 1;
}
// Get the file size
streamsize file_size = file.tellg();
file.seekg(0, ios::beg);
// Read the file content
stringstream file_content;
file_content << file.rdbuf();
file.close();
// Set the HTTP headers
struct curl_slist* headers = NULL;
headers = curl_slist_append(headers, "Content-Type: image/jpeg");
// Set the HTTP POST data
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, file_content.str().c_str());
curl_easy_setopt(curl, CURLOPT_POSTFIELDSIZE_LARGE, file_size);
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, write_callback);
// Perform the HTTP POST request
CURLcode result = curl_easy_perform(curl);
if (result != CURLE_OK)
{
cerr << "Failed to perform HTTP request: " << curl_easy_strerror(result) << endl;
return 1;
}
// Clean up
curl_easy_cleanup(curl);
curl_slist_free_all(headers);
cout << "Image uploaded successfully" << endl;
return 0;
}
```
在上面的代码中,我们使用libcurl库来实现HTTP请求。首先,我们打开要上传的图片文件,并读取其内容。然后,我们设置HTTP请求的头文件和POST数据。最后,我们使用curl_easy_perform()函数来执行HTTP请求。如果请求成功,函数会返回CURLE_OK。
需要注意的是,服务器端需要能够接收二进制流的图片数据,并将其解析保存。具体实现可以参考不同的后端框架或者自己编写解析代码。
相关推荐
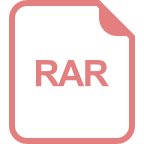












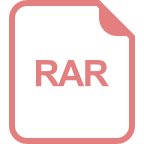