c++ 上传图片到http://169.254.1.10:8090/api/v1/~bali/ABC0123456789
时间: 2023-10-04 08:06:47 浏览: 158
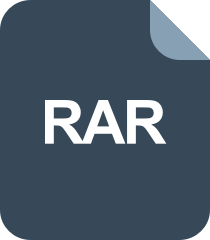
基于C++图片上传工具

你可以使用curl库来实现上传图片到指定的HTTP地址。下面是一个基本的上传代码示例:
```cpp
#include <curl/curl.h>
#include <iostream>
#include <fstream>
int main() {
CURL *curl;
CURLcode res;
// 读取图片数据
std::ifstream image_file("test.jpg", std::ios::binary | std::ios::ate);
std::streamsize image_size = image_file.tellg();
image_file.seekg(0, std::ios::beg);
char *image_data = new char[image_size];
if (!image_file.read(image_data, image_size)) {
std::cerr << "Failed to read image file." << std::endl;
return 1;
}
image_file.close();
// 初始化curl
curl = curl_easy_init();
if (!curl) {
std::cerr << "Failed to initialize curl." << std::endl;
return 1;
}
// 设置POST请求
curl_easy_setopt(curl, CURLOPT_POST, 1L);
// 设置请求地址
curl_easy_setopt(curl, CURLOPT_URL, "http://169.254.1.10:8090/api/v1/~bali/ABC0123456789");
// 设置请求数据
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, image_data);
curl_easy_setopt(curl, CURLOPT_POSTFIELDSIZE, static_cast<long>(image_size));
// 发送请求
res = curl_easy_perform(curl);
// 检查请求是否成功
if (res != CURLE_OK) {
std::cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << std::endl;
}
// 清理curl
curl_easy_cleanup(curl);
delete[] image_data;
return 0;
}
```
上述代码中,我们首先读取了一个名为test.jpg的图片文件,并将其存储在一个char数组中。然后,我们使用curl_easy_init函数初始化了一个CURL句柄,并通过curl_easy_setopt函数设置了POST请求和请求地址。接着,我们通过curl_easy_setopt函数设置了请求数据(即图片数据),并使用curl_easy_perform函数发送请求。最后,我们清理了CURL句柄并释放了图片数据的内存。
需要注意的是,上述示例仅提供了一个基本的上传代码框架,你需要根据实际情况进行修改和扩展。另外,如果你的图片数据很大,可能会导致内存不足,此时你需要将数据分批上传,或者使用内存映射等技术来处理。
阅读全文
相关推荐
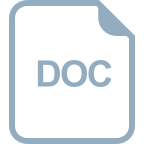















