用C语言表示:一个单链表L=(a1 , a2 , … , an-1 , an),其逆单链表定义为L’=( an , an-1 , … , a2 , a1),编写算法将单链表L逆置,要求逆单链表仍占用原单链表的空间。
时间: 2024-05-10 17:17:18 浏览: 114
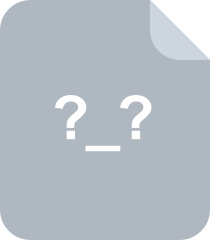
写一个算法将一单链表逆置。要求操作在原链表上进行。
以下是用C语言实现将单链表逆置并占用原单链表空间的算法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建单链表
Node* createLinkedList(int n) {
Node* head = NULL;
Node* tail = NULL;
for (int i = 0; i < n; i++) {
int x;
scanf("%d", &x);
Node* node = (Node*)malloc(sizeof(Node));
node->data = x;
node->next = NULL;
if (tail == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
// 将单链表逆置
void reverseLinkedList(Node* head) {
if (head == NULL || head->next == NULL) {
return;
}
Node* prev = NULL;
Node* curr = head;
Node* next = head->next;
while (next != NULL) {
curr->next = prev;
prev = curr;
curr = next;
next = next->next;
}
curr->next = prev;
}
// 打印单链表
void printLinkedList(Node* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
int n;
scanf("%d", &n);
Node* head = createLinkedList(n);
reverseLinkedList(head);
printLinkedList(head);
return 0;
}
```
例如,当输入单链表 1 -> 2 -> 3 -> 4 -> 5 时,程序将输出 5 -> 4 -> 3 -> 2 -> 1。
阅读全文
相关推荐









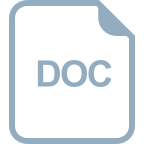





