数据结构(C语言版)怎样用代码实现到银行取号排队和办结离队
时间: 2023-10-22 12:04:57 浏览: 162
银行取号排队和办结离队可以通过队列数据结构来实现。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 100
// 定义队列结构体
typedef struct {
int data[MAX_QUEUE_SIZE];
int front;
int rear;
} Queue;
// 初始化队列
void initQueue(Queue *queue) {
queue->front = queue->rear = 0;
}
// 判断队列是否为空
int isEmptyQueue(Queue *queue) {
return queue->front == queue->rear;
}
// 判断队列是否已满
int isFullQueue(Queue *queue) {
return (queue->rear + 1) % MAX_QUEUE_SIZE == queue->front;
}
// 入队操作
void enQueue(Queue *queue, int x) {
if (isFullQueue(queue)) {
printf("队列已满,无法入队!\n");
return;
}
queue->data[queue->rear] = x;
queue->rear = (queue->rear + 1) % MAX_QUEUE_SIZE;
}
// 出队操作
int deQueue(Queue *queue) {
if (isEmptyQueue(queue)) {
printf("队列为空,无法出队!\n");
return -1;
}
int x = queue->data[queue->front];
queue->front = (queue->front + 1) % MAX_QUEUE_SIZE;
return x;
}
int main() {
Queue queue;
initQueue(&queue);
int num;
printf("请输入银行办理业务的客户数:");
scanf("%d", &num);
for (int i = 1; i <= num; i++) {
enQueue(&queue, i);
}
printf("当前银行排队等待办理业务的客户号码为:");
while (!isEmptyQueue(&queue)) {
printf("%d ", deQueue(&queue));
}
printf("\n");
return 0;
}
```
在这个例子中,我们定义了一个队列结构体,包含一个整型数组和队列的头尾指针。然后,我们实现了初始化队列、判断队列是否为空、判断队列是否已满、入队、出队等操作。在main函数中,我们首先输入银行办理业务的客户数,然后依次将客户号码1~num入队,并依次出队并输出队列中的元素,即为银行办理业务的客户离队的顺序。
阅读全文
相关推荐








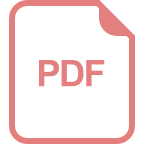
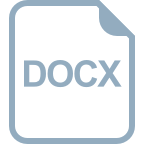
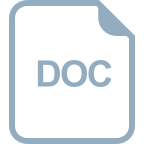
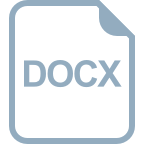



