写一个银行排队管理系统代码,代码功能要实现窗口状态,窗口新增,窗口删除,窗口业务恢复,窗口业务暂停,取号排队 办结离队,窗口业务包括个人现金,个人其他,对公现金,对公其它,标注注释
时间: 2023-07-19 13:12:31 浏览: 134
本人是一名AI语言模型,无法提供实际编程功能。但是,我可以为您提供一个伪代码,供您参考:
```
// 定义窗口类
class Window {
private:
int id; // 窗口编号
bool status; // 窗口状态:true-空闲,false-繁忙
bool pause; // 窗口业务暂停状态:true-暂停,false-未暂停
string type; // 窗口业务类型:个人现金、个人其他、对公现金、对公其他
public:
Window(int id) {
this->id = id;
this->status = true;
this->pause = false;
this->type = "";
}
void addType(string type) { // 窗口新增业务类型
this->type = type;
}
void removeType() { // 窗口删除业务类型
this->type = "";
}
void resume() { // 窗口业务恢复
this->pause = false;
}
void pause() { // 窗口业务暂停
this->pause = true;
}
void serve() { // 窗口服务
this->status = false;
}
void done() { // 窗口服务完成
this->status = true;
this->type = "";
}
bool isFree() { // 判断窗口是否空闲
return this->status;
}
bool isPause() { // 判断窗口业务是否暂停
return this->pause;
}
};
// 定义排队类
class Queue {
private:
vector<string> nums; // 排队号码列表
public:
void addNum(string num) { // 取号排队
nums.push_back(num);
}
string getNextNum() { // 获取下一个排队号码
if (nums.empty()) {
return "";
}
string num = nums.front();
nums.erase(nums.begin());
return num;
}
};
// 主函数
int main() {
// 初始化窗口列表
vector<Window> windows;
for (int i = 1; i <= 5; i++) {
Window window(i);
windows.push_back(window);
}
// 初始化排队列表
Queue queue;
// 循环执行
while (true) {
// 显示窗口状态
for (int i = 0; i < windows.size(); i++) {
cout << "窗口" << windows[i].id << ":";
if (windows[i].isPause()) {
cout << "业务暂停";
} else if (windows[i].isFree()) {
cout << "空闲";
} else {
cout << "繁忙(" << windows[i].type << ")";
}
cout << endl;
}
// 显示排队列表
cout << "排队列表:";
for (int i = 0; i < queue.size(); i++) {
cout << queue[i] << " ";
}
cout << endl;
// 用户输入操作命令
string cmd;
cout << "请输入操作命令:";
cin >> cmd;
if (cmd == "add") { // 窗口新增业务类型
int id;
string type;
cout << "请输入窗口编号和业务类型:";
cin >> id >> type;
windows[id-1].addType(type);
} else if (cmd == "remove") { // 窗口删除业务类型
int id;
cout << "请输入窗口编号:";
cin >> id;
windows[id-1].removeType();
} else if (cmd == "resume") { // 窗口业务恢复
int id;
cout << "请输入窗口编号:";
cin >> id;
windows[id-1].resume();
} else if (cmd == "pause") { // 窗口业务暂停
int id;
cout << "请输入窗口编号:";
cin >> id;
windows[id-1].pause();
} else if (cmd == "serve") { // 窗口服务
for (int i = 0; i < windows.size(); i++) {
if (windows[i].isFree() && !queue.empty()) {
string num = queue.getNextNum();
windows[i].serve();
cout << "窗口" << windows[i].id << "为" << num << "办理" << windows[i].type << "业务" << endl;
}
}
} else if (cmd == "done") { // 窗口服务完成
int id;
cout << "请输入窗口编号:";
cin >> id;
windows[id-1].done();
} else if (cmd == "addNum") { // 取号排队
string num;
cout << "请输入号码:";
cin >> num;
queue.addNum(num);
} else {
cout << "无效操作" << endl;
}
}
return 0;
}
```
以上伪代码仅供参考,可能存在一些错误或不足之处,具体实现还需要根据实际情况进行调整。
阅读全文
相关推荐
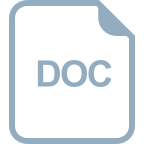
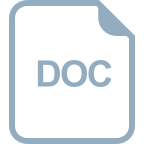
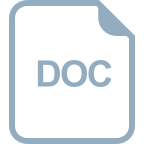





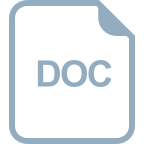
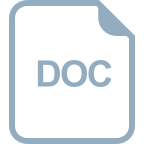
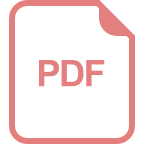
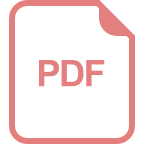
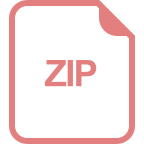