python通过图像交并比计算AP
时间: 2023-07-12 19:00:21 浏览: 120
首先,AP (Average Precision) 是一种用于评估目标检测算法性能的指标。计算AP需要先计算每个类别的Precision-Recall曲线,然后再计算曲线下面积 (Area Under Curve, AUC)。
图像交并比 (Intersection over Union, IoU) 是计算目标检测算法输出结果与真实标注框之间重合程度的一种常用指标。在计算AP时,我们可以根据IoU阈值来判断一个检测结果是否正确。例如,当IoU阈值为0.5时,如果检测结果与真实标注框的IoU值大于0.5,则认为该结果正确。
下面是Python代码示例,可以用来计算一个类别的AP,并且使用IoU阈值为0.5:
```python
import numpy as np
def compute_iou(bbox1, bbox2):
"""
计算两个bbox之间的IoU
bbox1, bbox2: [x1, y1, x2, y2]
"""
x1 = max(bbox1[0], bbox2[0])
y1 = max(bbox1[1], bbox2[1])
x2 = min(bbox1[2], bbox2[2])
y2 = min(bbox1[3], bbox2[3])
inter_area = max(0, x2 - x1 + 1) * max(0, y2 - y1 + 1)
bbox1_area = (bbox1[2] - bbox1[0] + 1) * (bbox1[3] - bbox1[1] + 1)
bbox2_area = (bbox2[2] - bbox2[0] + 1) * (bbox2[3] - bbox2[1] + 1)
iou = inter_area / float(bbox1_area + bbox2_area - inter_area)
return iou
def compute_ap(gt_bboxes, pred_bboxes, iou_thres=0.5):
"""
计算单个类别的AP
gt_bboxes: 真实标注框集合,格式为[[x1, y1, x2, y2], ...]
pred_bboxes: 预测框集合,格式为[[x1, y1, x2, y2, score], ...]
iou_thres: IoU阈值,默认为0.5
"""
# 按照预测框的score从大到小排序
sorted_pred_bboxes = sorted(pred_bboxes, key=lambda x: x[4], reverse=True)
# 初始化Precision-Recall
tp = np.zeros(len(sorted_pred_bboxes))
fp = np.zeros(len(sorted_pred_bboxes))
num_gt_bboxes = len(gt_bboxes)
# 对每个预测框计算Precision-Recall
for i, pred_bbox in enumerate(sorted_pred_bboxes):
if len(gt_bboxes) == 0:
fp[i] = 1
continue
iou_list = [compute_iou(gt_bbox, pred_bbox[:4]) for gt_bbox in gt_bboxes]
max_iou_idx = np.argmax(iou_list)
if iou_list[max_iou_idx] >= iou_thres:
if tp[max_iou_idx] == 0: # 该GT框还未匹配
tp[i] = 1
gt_bboxes.pop(max_iou_idx)
else: # 该GT框已经匹配
fp[i] = 1
else:
fp[i] = 1
# 计算Precision-Recall曲线和AP
tp = np.cumsum(tp)
fp = np.cumsum(fp)
recall = tp / float(num_gt_bboxes)
precision = tp / np.maximum(tp + fp, 1e-6)
ap = compute_ap_from_pr(precision, recall)
return ap
def compute_ap_from_pr(precision, recall):
"""
计算Precision-Recall曲线下面积
"""
mrec = np.concatenate(([0.], recall, [1.]))
mpre = np.concatenate(([0.], precision, [0.]))
# 将Precision按照Recall从大到小排序
for i in range(len(mpre) - 2, -1, -1):
mpre[i] = max(mpre[i], mpre[i + 1])
i = np.where(mrec[1:] != mrec[:-1])[0]
ap = np.sum((mrec[i + 1] - mrec[i]) * mpre[i + 1])
return ap
```
该代码假定真实标注框和预测框都是矩形框,格式为[x1, y1, x2, y2]。其中,x1, y1是左上角坐标,x2, y2是右下角坐标。预测框还需要包含一个score值,表示预测框的置信度。该代码返回单个类别的AP值。
阅读全文
相关推荐
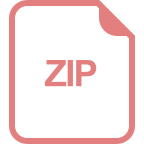
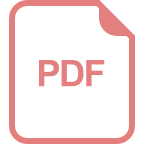
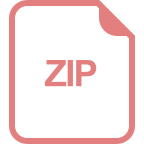
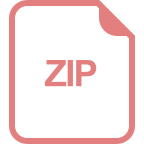
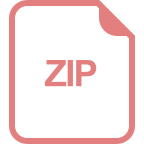
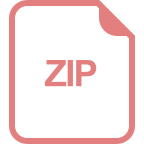
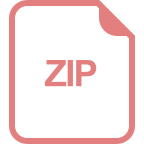
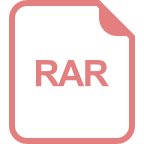
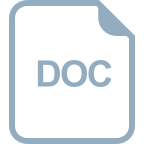
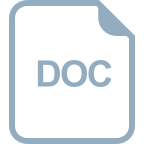
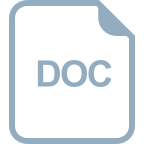
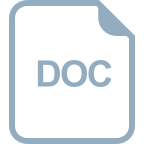
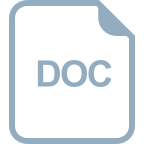
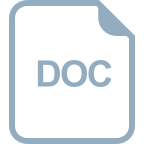
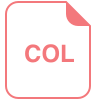
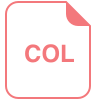

